mirror of
https://github.com/RGBCube/serenity
synced 2025-07-16 20:47:34 +00:00
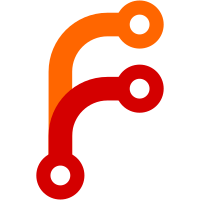
Previously, we determined the positions of glyphs for each text run at the time of painting, which constituted a significant portion of the painting process according to profiles. However, since we already go through each glyph to figure out the width of each fragment during layout, we can simultaneously gather data about the position of each glyph in the layout phase and utilize this information in the painting phase. I had to update expectations for a couple of reference tests. These updates are due to the fact that we now measure glyph positions during layout using a 1x font, and then linearly scale each glyph's position to device pixels during painting. This approach should be acceptable, considering we measure a fragment's width and height with an unscaled font during layout.
70 lines
2.1 KiB
C++
70 lines
2.1 KiB
C++
/*
|
|
* Copyright (c) 2022, Andreas Kling <kling@serenityos.org>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <LibWeb/Layout/InlineFormattingContext.h>
|
|
|
|
namespace Web::Layout {
|
|
|
|
class LineBuilder {
|
|
AK_MAKE_NONCOPYABLE(LineBuilder);
|
|
AK_MAKE_NONMOVABLE(LineBuilder);
|
|
|
|
public:
|
|
LineBuilder(InlineFormattingContext&, LayoutState&);
|
|
~LineBuilder();
|
|
|
|
enum class ForcedBreak {
|
|
No,
|
|
Yes,
|
|
};
|
|
|
|
void break_line(ForcedBreak, Optional<CSSPixels> next_item_width = {});
|
|
void append_box(Box const&, CSSPixels leading_size, CSSPixels trailing_size, CSSPixels leading_margin, CSSPixels trailing_margin);
|
|
void append_text_chunk(TextNode const&, size_t offset_in_node, size_t length_in_node, CSSPixels leading_size, CSSPixels trailing_size, CSSPixels leading_margin, CSSPixels trailing_margin, CSSPixels content_width, CSSPixels content_height, Span<Gfx::DrawGlyphOrEmoji>);
|
|
|
|
// Returns whether a line break occurred.
|
|
bool break_if_needed(CSSPixels next_item_width)
|
|
{
|
|
if (should_break(next_item_width)) {
|
|
break_line(LineBuilder::ForcedBreak::No, next_item_width);
|
|
return true;
|
|
}
|
|
return false;
|
|
}
|
|
|
|
void update_last_line();
|
|
|
|
void remove_last_line_if_empty();
|
|
|
|
CSSPixels current_y() const { return m_current_y; }
|
|
void set_current_y(CSSPixels y) { m_current_y = y; }
|
|
|
|
void recalculate_available_space();
|
|
CSSPixels y_for_float_to_be_inserted_here(Box const&);
|
|
|
|
auto& inline_formatting_context() { return m_context; }
|
|
|
|
private:
|
|
void begin_new_line(bool increment_y, bool is_first_break_in_sequence = true);
|
|
|
|
bool should_break(CSSPixels next_item_width);
|
|
|
|
LineBox& ensure_last_line_box();
|
|
|
|
InlineFormattingContext& m_context;
|
|
LayoutState& m_layout_state;
|
|
LayoutState::UsedValues& m_containing_block_state;
|
|
AvailableSize m_available_width_for_current_line { AvailableSize::make_indefinite() };
|
|
CSSPixels m_current_y { 0 };
|
|
CSSPixels m_max_height_on_current_line { 0 };
|
|
CSSPixels m_text_indent { 0 };
|
|
|
|
bool m_last_line_needs_update { false };
|
|
};
|
|
|
|
}
|