mirror of
https://github.com/RGBCube/serenity
synced 2025-05-14 08:14:58 +00:00
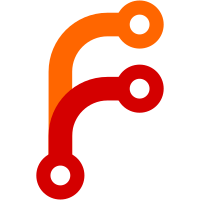
Rather than try to lay out masks normally, this updates the TreeBuilder to create layout nodes for masks as a child of their user (i.e. the masked element). This allows each use of a mask to be laid out differently, which makes supporting `maskContentUnits=objectBoundingBox` fairly easy. The `SVGFormattingContext` is then updated to lay out masks last (as their sizing may depend on their parent), and treats them like viewports. This is pretty ad-hoc, but the SVG specification does not give any guidance on how to actually implement this.
72 lines
2.4 KiB
C++
72 lines
2.4 KiB
C++
/*
|
|
* Copyright (c) 2022, Andreas Kling <kling@serenityos.org>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <LibWeb/Layout/SVGGraphicsBox.h>
|
|
#include <LibWeb/Painting/SVGPaintable.h>
|
|
|
|
namespace Web::Painting {
|
|
|
|
class SVGGraphicsPaintable : public SVGPaintable {
|
|
JS_CELL(SVGGraphicsPaintable, SVGPaintable);
|
|
|
|
public:
|
|
class ComputedTransforms {
|
|
public:
|
|
ComputedTransforms(Gfx::AffineTransform svg_to_viewbox_transform, Gfx::AffineTransform svg_transform)
|
|
: m_svg_to_viewbox_transform(svg_to_viewbox_transform)
|
|
, m_svg_transform(svg_transform)
|
|
{
|
|
}
|
|
|
|
ComputedTransforms() = default;
|
|
|
|
Gfx::AffineTransform const& svg_to_viewbox_transform() const { return m_svg_to_viewbox_transform; }
|
|
Gfx::AffineTransform const& svg_transform() const { return m_svg_transform; }
|
|
|
|
Gfx::AffineTransform svg_to_css_pixels_transform(
|
|
Optional<Gfx::AffineTransform const&> additional_svg_transform = {}) const
|
|
{
|
|
return Gfx::AffineTransform {}.multiply(svg_to_viewbox_transform()).multiply(additional_svg_transform.value_or(Gfx::AffineTransform {})).multiply(svg_transform());
|
|
}
|
|
|
|
Gfx::AffineTransform svg_to_device_pixels_transform(PaintContext const& context) const
|
|
{
|
|
auto css_scale = context.device_pixels_per_css_pixel();
|
|
return Gfx::AffineTransform {}.scale({ css_scale, css_scale }).multiply(svg_to_css_pixels_transform(context.svg_transform()));
|
|
}
|
|
|
|
private:
|
|
Gfx::AffineTransform m_svg_to_viewbox_transform {};
|
|
Gfx::AffineTransform m_svg_transform {};
|
|
};
|
|
|
|
static JS::NonnullGCPtr<SVGGraphicsPaintable> create(Layout::SVGGraphicsBox const&);
|
|
|
|
Layout::SVGGraphicsBox const& layout_box() const;
|
|
|
|
virtual Optional<CSSPixelRect> get_masking_area() const override;
|
|
virtual Optional<Gfx::Bitmap::MaskKind> get_mask_type() const override;
|
|
virtual RefPtr<Gfx::Bitmap> calculate_mask(PaintContext&, CSSPixelRect const& masking_area) const override;
|
|
|
|
void set_computed_transforms(ComputedTransforms computed_transforms)
|
|
{
|
|
m_computed_transforms = computed_transforms;
|
|
}
|
|
|
|
ComputedTransforms const& computed_transforms() const
|
|
{
|
|
return m_computed_transforms;
|
|
}
|
|
|
|
protected:
|
|
SVGGraphicsPaintable(Layout::SVGGraphicsBox const&);
|
|
|
|
ComputedTransforms m_computed_transforms;
|
|
};
|
|
|
|
}
|