mirror of
https://github.com/RGBCube/serenity
synced 2025-05-14 07:54:58 +00:00
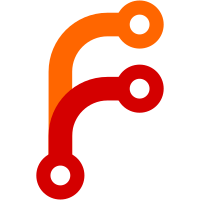
In this commit we have optimized the handling of scroll offsets and clip rectangles to improve performance. Previously, the process involved multiple full traversals of the paintable tree before each repaint, which was highly inefficient, especially on pages with a large number of paintables. The steps were: 1. Traverse the paintable tree to identify all boxes with scrollable or clipped overflow. 2. Gather the accumulated scroll offset or clip rectangle for each box. 3. Perform another traversal to apply the corresponding scroll offset and clip rectangle to each paintable. To address this, we've adopted a new strategy that separates the assignment of the scroll/clip frame from the refresh of accumulated scroll offsets and clip rectangles, thus reducing the workload: 1. Post-relayout: Identify all boxes with overflow and link each paintable to the state of its containing scroll/clip frame. 2. Pre-repaint: Update the clip rectangle and scroll offset only in the previously identified boxes. This adjustment ensures that the costly tree traversals are only necessary after a relayout, substantially decreasing the amount of work required before each repaint.
39 lines
952 B
C++
39 lines
952 B
C++
/*
|
|
* Copyright (c) 2023, Andreas Kling <kling@serenityos.org>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <LibWeb/Painting/PaintableBox.h>
|
|
|
|
namespace Web::Painting {
|
|
|
|
class ViewportPaintable final : public PaintableWithLines {
|
|
JS_CELL(ViewportPaintable, PaintableWithLines);
|
|
|
|
public:
|
|
static JS::NonnullGCPtr<ViewportPaintable> create(Layout::Viewport const&);
|
|
virtual ~ViewportPaintable() override;
|
|
|
|
void paint_all_phases(PaintContext&);
|
|
void build_stacking_context_tree_if_needed();
|
|
|
|
HashMap<PaintableBox const*, RefPtr<ScrollFrame>> scroll_state;
|
|
void assign_scroll_frames();
|
|
void refresh_scroll_state();
|
|
|
|
HashMap<PaintableBox const*, RefPtr<ClipFrame>> clip_state;
|
|
void assign_clip_frames();
|
|
void refresh_clip_state();
|
|
|
|
void resolve_paint_only_properties();
|
|
|
|
private:
|
|
void build_stacking_context_tree();
|
|
|
|
explicit ViewportPaintable(Layout::Viewport const&);
|
|
};
|
|
|
|
}
|