mirror of
https://github.com/RGBCube/serenity
synced 2025-07-03 00:52:12 +00:00
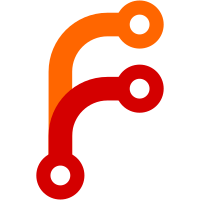
In this change, updating layout and painting are moved to the EventLoop processing steps. This modification allows the addition of resize observation dispatching that needs to happen in event loop processing steps and must occur in the following order relative to layout and painting: 1. Update layout. 2. Gather and broadcast resize observations. 3. Paint.
46 lines
1.3 KiB
C++
46 lines
1.3 KiB
C++
/*
|
|
* Copyright (c) 2023, Andrew Kaster <akaster@serenityos.org>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <LibGfx/Rect.h>
|
|
#include <LibWeb/Page/Page.h>
|
|
#include <LibWeb/PixelUnits.h>
|
|
#include <WebWorker/Forward.h>
|
|
|
|
namespace WebWorker {
|
|
|
|
class PageHost final : public Web::PageClient {
|
|
JS_CELL(PageHost, Web::PageClient);
|
|
|
|
public:
|
|
static JS::NonnullGCPtr<PageHost> create(JS::VM& vm, ConnectionFromClient& client);
|
|
|
|
virtual ~PageHost();
|
|
|
|
virtual Web::Page& page() override;
|
|
virtual Web::Page const& page() const override;
|
|
virtual bool is_connection_open() const override;
|
|
virtual Gfx::Palette palette() const override;
|
|
virtual Web::DevicePixelRect screen_rect() const override;
|
|
virtual double device_pixels_per_css_pixel() const override;
|
|
virtual Web::CSS::PreferredColorScheme preferred_color_scheme() const override;
|
|
virtual void paint(Web::DevicePixelRect const&, Gfx::Bitmap&, Web::PaintOptions = {}) override;
|
|
virtual void request_file(Web::FileRequest) override;
|
|
virtual void schedule_repaint() override {};
|
|
|
|
private:
|
|
explicit PageHost(ConnectionFromClient&);
|
|
virtual void visit_edges(JS::Cell::Visitor&) override;
|
|
|
|
void setup_palette();
|
|
|
|
ConnectionFromClient& m_client;
|
|
JS::NonnullGCPtr<Web::Page> m_page;
|
|
RefPtr<Gfx::PaletteImpl> m_palette_impl;
|
|
};
|
|
|
|
}
|