mirror of
https://github.com/RGBCube/serenity
synced 2025-07-03 10:22:06 +00:00
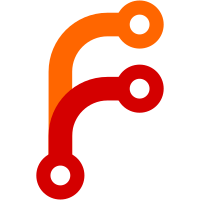
With this change, we now have ~1200 CellAllocators across both LibJS and LibWeb in a normal WebContent instance. This gives us a minimum heap size of 4.7 MiB in the scenario where we only have one cell allocated per type. Of course, in practice there will be many more of each type, so the effective overhead is quite a bit smaller than that in practice. I left a few types unconverted to this mechanism because I got tired of doing this. :^)
44 lines
1.6 KiB
C++
44 lines
1.6 KiB
C++
/*
|
|
* Copyright (c) 2023, Matthew Olsson <mattco@serenityos.org>.
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <LibWeb/Animations/AnimationTimeline.h>
|
|
#include <LibWeb/HighResolutionTime/DOMHighResTimeStamp.h>
|
|
#include <LibWeb/WebIDL/ExceptionOr.h>
|
|
|
|
namespace Web::Animations {
|
|
|
|
// https://www.w3.org/TR/web-animations-1/#dictdef-documenttimelineoptions
|
|
struct DocumentTimelineOptions {
|
|
HighResolutionTime::DOMHighResTimeStamp origin_time { 0.0 };
|
|
};
|
|
|
|
// https://www.w3.org/TR/web-animations-1/#the-documenttimeline-interface
|
|
class DocumentTimeline : public AnimationTimeline {
|
|
WEB_PLATFORM_OBJECT(DocumentTimeline, AnimationTimeline);
|
|
JS_DECLARE_ALLOCATOR(DocumentTimeline);
|
|
|
|
public:
|
|
static JS::NonnullGCPtr<DocumentTimeline> create(JS::Realm&, DOM::Document&, HighResolutionTime::DOMHighResTimeStamp origin_time);
|
|
static WebIDL::ExceptionOr<JS::NonnullGCPtr<DocumentTimeline>> construct_impl(JS::Realm&, DocumentTimelineOptions options = {});
|
|
|
|
virtual WebIDL::ExceptionOr<void> set_current_time(Optional<double> current_time) override;
|
|
virtual bool is_inactive() const override;
|
|
|
|
virtual Optional<double> convert_a_timeline_time_to_an_original_relative_time(Optional<double>) override;
|
|
virtual bool can_convert_a_timeline_time_to_an_original_relative_time() const override { return true; }
|
|
|
|
private:
|
|
DocumentTimeline(JS::Realm&, DOM::Document&, HighResolutionTime::DOMHighResTimeStamp origin_time);
|
|
virtual ~DocumentTimeline() override = default;
|
|
|
|
virtual void initialize(JS::Realm&) override;
|
|
|
|
HighResolutionTime::DOMHighResTimeStamp m_origin_time;
|
|
};
|
|
|
|
}
|