mirror of
https://github.com/RGBCube/serenity
synced 2025-07-14 21:17:35 +00:00
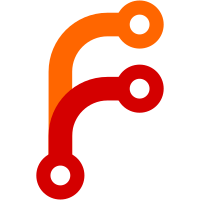
This commit introduces NamedVariableDeclaration and SSAVariableDeclaration and allows storing both of them in Variable node. Also, it adds additional structures in FunctionDefinition and BasicBlock, which will be used to store SSA form related information.
42 lines
1,022 B
C++
42 lines
1,022 B
C++
/*
|
|
* Copyright (c) 2023, Dan Klishch <danilklishch@gmail.com>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#include "Function.h"
|
|
#include "AST/AST.h"
|
|
#include "Compiler/ControlFlowGraph.h"
|
|
|
|
namespace JSSpecCompiler {
|
|
|
|
FunctionDefinitionRef TranslationUnit::adopt_function(NonnullRefPtr<FunctionDefinition>&& function)
|
|
{
|
|
function->m_translation_unit = this;
|
|
function_index.set(function->m_name, make_ref_counted<FunctionPointer>(function));
|
|
|
|
FunctionDefinitionRef result = function.ptr();
|
|
function_definitions.append(move(function));
|
|
return result;
|
|
}
|
|
|
|
FunctionDeclaration::FunctionDeclaration(StringView name)
|
|
: m_name(name)
|
|
{
|
|
}
|
|
|
|
FunctionDefinition::FunctionDefinition(StringView name, Tree ast)
|
|
: FunctionDeclaration(name)
|
|
, m_ast(move(ast))
|
|
, m_return_value(make_ref_counted<NamedVariableDeclaration>("$return"sv))
|
|
{
|
|
}
|
|
|
|
void FunctionDefinition::reindex_ssa_variables()
|
|
{
|
|
size_t index = 0;
|
|
for (auto const& var : m_local_ssa_variables)
|
|
var->m_index = index++;
|
|
}
|
|
|
|
}
|