mirror of
https://github.com/RGBCube/serenity
synced 2025-05-21 23:25:07 +00:00
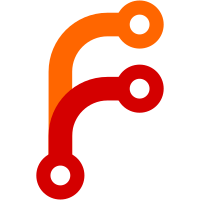
Currently, we create a new SQL::Database object for each database we are requested to open. When multiple clients connect to the same database, the same underlying database file is opened and cached each time. This results in updates from one client not being propagated to others. To prevent this, when a database is requested to be open, check if it is already open. We can then re-use that SQL::Database object for the new connection.
42 lines
1.3 KiB
C++
42 lines
1.3 KiB
C++
/*
|
|
* Copyright (c) 2021, Jan de Visser <jan@de-visser.net>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <AK/NonnullRefPtr.h>
|
|
#include <LibCore/Object.h>
|
|
#include <LibSQL/Database.h>
|
|
#include <LibSQL/Result.h>
|
|
#include <LibSQL/Type.h>
|
|
#include <SQLServer/Forward.h>
|
|
|
|
namespace SQLServer {
|
|
|
|
class DatabaseConnection final : public Core::Object {
|
|
C_OBJECT_ABSTRACT(DatabaseConnection)
|
|
|
|
public:
|
|
static ErrorOr<NonnullRefPtr<DatabaseConnection>> create(StringView database_path, DeprecatedString database_name, int client_id);
|
|
~DatabaseConnection() override = default;
|
|
|
|
static RefPtr<DatabaseConnection> connection_for(SQL::ConnectionID connection_id);
|
|
SQL::ConnectionID connection_id() const { return m_connection_id; }
|
|
int client_id() const { return m_client_id; }
|
|
NonnullRefPtr<SQL::Database> database() { return m_database; }
|
|
StringView database_name() const { return m_database_name; }
|
|
void disconnect();
|
|
SQL::ResultOr<SQL::StatementID> prepare_statement(StringView sql);
|
|
|
|
private:
|
|
DatabaseConnection(NonnullRefPtr<SQL::Database> database, DeprecatedString database_name, int client_id);
|
|
|
|
NonnullRefPtr<SQL::Database> m_database;
|
|
DeprecatedString m_database_name;
|
|
SQL::ConnectionID m_connection_id { 0 };
|
|
int m_client_id { 0 };
|
|
};
|
|
|
|
}
|