mirror of
https://github.com/RGBCube/serenity
synced 2025-07-05 21:47:36 +00:00
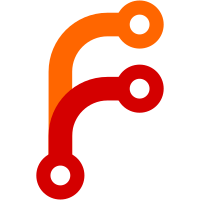
Not a huge deal because the base MediaPaintable class goes very out of its way to paint within the confines of its own box, but just to be safe, this was missed when adding the AudioPaintable class.
71 lines
2.1 KiB
C++
71 lines
2.1 KiB
C++
/*
|
|
* Copyright (c) 2023, Tim Flynn <trflynn89@serenityos.org>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#include <AK/Array.h>
|
|
#include <AK/NumberFormat.h>
|
|
#include <LibGUI/Event.h>
|
|
#include <LibGfx/AntiAliasingPainter.h>
|
|
#include <LibWeb/DOM/Document.h>
|
|
#include <LibWeb/HTML/AudioTrackList.h>
|
|
#include <LibWeb/HTML/HTMLAudioElement.h>
|
|
#include <LibWeb/HTML/HTMLMediaElement.h>
|
|
#include <LibWeb/Layout/AudioBox.h>
|
|
#include <LibWeb/Painting/AudioPaintable.h>
|
|
#include <LibWeb/Painting/BorderRadiusCornerClipper.h>
|
|
|
|
namespace Web::Painting {
|
|
|
|
JS::NonnullGCPtr<AudioPaintable> AudioPaintable::create(Layout::AudioBox const& layout_box)
|
|
{
|
|
return layout_box.heap().allocate_without_realm<AudioPaintable>(layout_box);
|
|
}
|
|
|
|
AudioPaintable::AudioPaintable(Layout::AudioBox const& layout_box)
|
|
: MediaPaintable(layout_box)
|
|
{
|
|
}
|
|
|
|
Layout::AudioBox& AudioPaintable::layout_box()
|
|
{
|
|
return static_cast<Layout::AudioBox&>(layout_node());
|
|
}
|
|
|
|
Layout::AudioBox const& AudioPaintable::layout_box() const
|
|
{
|
|
return static_cast<Layout::AudioBox const&>(layout_node());
|
|
}
|
|
|
|
void AudioPaintable::paint(PaintContext& context, PaintPhase phase) const
|
|
{
|
|
if (!is_visible())
|
|
return;
|
|
|
|
// FIXME: This should be done at a different level.
|
|
if (is_out_of_view(context))
|
|
return;
|
|
|
|
Base::paint(context, phase);
|
|
|
|
if (phase != PaintPhase::Foreground)
|
|
return;
|
|
|
|
Gfx::PainterStateSaver saver { context.painter() };
|
|
|
|
auto audio_rect = context.rounded_device_rect(absolute_rect());
|
|
context.painter().add_clip_rect(audio_rect.to_type<int>());
|
|
|
|
ScopedCornerRadiusClip corner_clip { context, context.painter(), audio_rect, normalized_border_radii_data(ShrinkRadiiForBorders::Yes) };
|
|
|
|
auto const& audio_element = layout_box().dom_node();
|
|
auto mouse_position = MediaPaintable::mouse_position(context, audio_element);
|
|
|
|
auto paint_user_agent_controls = audio_element.has_attribute(HTML::AttributeNames::controls) || audio_element.is_scripting_disabled();
|
|
|
|
if (paint_user_agent_controls)
|
|
paint_media_controls(context, audio_element, audio_rect, mouse_position);
|
|
}
|
|
|
|
}
|