mirror of
https://github.com/RGBCube/serenity
synced 2025-07-12 19:07:35 +00:00
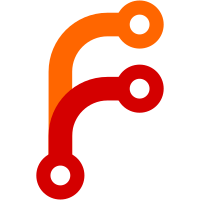
The problem was a bit more complex than originally anticipated, and the root of the problem is that the "coordinates" of a pixel are actually the top left of the pixel, and when we're really zoomed in, the difference in editor coordinates of the top-left and the center of the pixel is significant. So, we need to offset the "start" point when we are painting on the editor to account for this, based on the current scale. This patch adds a `editor_stroke_position` in `Tool` which can be used to compute what point (in editor coords) we should use for a given pixel and it's corresponding stroke thickness. Note that this doesn't really work well with the ellipse, since that is drawn with a different mechanism. Using this new method with the `EllipseTool` seems to give the same (or slightly worse) results, so I have not changed anything there for now.
64 lines
1.5 KiB
C++
64 lines
1.5 KiB
C++
/*
|
|
* Copyright (c) 2018-2020, Andreas Kling <kling@serenityos.org>
|
|
* Copyright (c) 2021, Mustafa Quraish <mustafa@cs.toronto.edu>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#include "Tool.h"
|
|
#include "ImageEditor.h"
|
|
#include <LibGUI/Action.h>
|
|
|
|
namespace PixelPaint {
|
|
|
|
Tool::Tool()
|
|
{
|
|
}
|
|
|
|
Tool::~Tool()
|
|
{
|
|
}
|
|
|
|
void Tool::setup(ImageEditor& editor)
|
|
{
|
|
m_editor = editor;
|
|
}
|
|
|
|
void Tool::set_action(GUI::Action* action)
|
|
{
|
|
m_action = action;
|
|
}
|
|
|
|
void Tool::on_keydown(GUI::KeyEvent& event)
|
|
{
|
|
switch (event.key()) {
|
|
case KeyCode::Key_LeftBracket:
|
|
if (m_primary_slider)
|
|
m_primary_slider->set_value(m_primary_slider->value() - 1);
|
|
break;
|
|
case KeyCode::Key_RightBracket:
|
|
if (m_primary_slider)
|
|
m_primary_slider->set_value(m_primary_slider->value() + 1);
|
|
break;
|
|
case KeyCode::Key_LeftBrace:
|
|
if (m_secondary_slider)
|
|
m_secondary_slider->set_value(m_secondary_slider->value() - 1);
|
|
break;
|
|
case KeyCode::Key_RightBrace:
|
|
if (m_secondary_slider)
|
|
m_secondary_slider->set_value(m_secondary_slider->value() + 1);
|
|
break;
|
|
default:
|
|
break;
|
|
}
|
|
}
|
|
|
|
Gfx::IntPoint Tool::editor_stroke_position(Gfx::IntPoint const& pixel_coords, int stroke_thickness) const
|
|
{
|
|
auto position = m_editor->image_position_to_editor_position(pixel_coords);
|
|
auto offset = (stroke_thickness % 2 == 0) ? m_editor->scale() : m_editor->scale() / 2;
|
|
position = position.translated(offset, offset);
|
|
return position.to_type<int>();
|
|
}
|
|
|
|
}
|