mirror of
https://github.com/RGBCube/serenity
synced 2025-05-14 14:24:57 +00:00
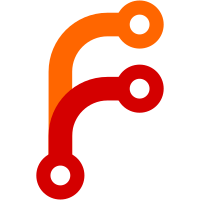
There are now 2 separate classes for almost the same object type: - EnumerableDeviceIdentifier, which is used in the enumeration code for all PCI host controller classes. This is allowed to be moved and copied, as it doesn't support ref-counting. - DeviceIdentifier, which inherits from EnumerableDeviceIdentifier. This class uses ref-counting, and is not allowed to be copied. It has a spinlock member in its structure to allow safely executing complicated IO sequences on a PCI device and its space configuration. There's a static method that allows a quick conversion from EnumerableDeviceIdentifier to DeviceIdentifier while creating a NonnullRefPtr out of it. The reason for doing this is for the sake of integrity and reliablity of the system in 2 places: - Ensure that "complicated" tasks that rely on manipulating PCI device registers are done in a safe manner. For example, determining a PCI BAR space size requires multiple read and writes to the same register, and if another CPU tries to do something else with our selected register, then the result will be a catastrophe. - Allow the PCI API to have a united form around a shared object which actually holds much more data than the PCI::Address structure. This is fundamental if we want to do certain types of optimizations, and be able to support more features of the PCI bus in the foreseeable future. This patch already has several implications: - All PCI::Device(s) hold a reference to a DeviceIdentifier structure being given originally from the PCI::Access singleton. This means that all instances of DeviceIdentifier structures are located in one place, and all references are pointing to that location. This ensures that locking the operation spinlock will take effect in all the appropriate places. - We no longer support adding PCI host controllers and then immediately allow for enumerating it with a lambda function. It was found that this method is extremely broken and too much complicated to work reliably with the new paradigm being introduced in this patch. This means that for Volume Management Devices (Intel VMD devices), we simply first enumerate the PCI bus for such devices in the storage code, and if we find a device, we attach it in the PCI::Access method which will scan for devices behind that bridge and will add new DeviceIdentifier(s) objects to its internal Vector. Afterwards, we just continue as usual with scanning for actual storage controllers, so we will find a corresponding NVMe controllers if there were any behind that VMD bridge.
280 lines
9.2 KiB
C++
280 lines
9.2 KiB
C++
/*
|
|
* Copyright (c) 2021, Liav A. <liavalb@hotmail.co.il>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#include <Kernel/Bus/PCI/API.h>
|
|
#include <Kernel/Bus/PCI/Access.h>
|
|
#include <Kernel/Sections.h>
|
|
|
|
namespace Kernel::PCI {
|
|
|
|
void write8_locked(DeviceIdentifier const& identifier, PCI::RegisterOffset field, u8 value)
|
|
{
|
|
VERIFY(identifier.operation_lock().is_locked());
|
|
Access::the().write8_field(identifier, to_underlying(field), value);
|
|
}
|
|
void write16_locked(DeviceIdentifier const& identifier, PCI::RegisterOffset field, u16 value)
|
|
{
|
|
VERIFY(identifier.operation_lock().is_locked());
|
|
Access::the().write16_field(identifier, to_underlying(field), value);
|
|
}
|
|
void write32_locked(DeviceIdentifier const& identifier, PCI::RegisterOffset field, u32 value)
|
|
{
|
|
VERIFY(identifier.operation_lock().is_locked());
|
|
Access::the().write32_field(identifier, to_underlying(field), value);
|
|
}
|
|
|
|
u8 read8_locked(DeviceIdentifier const& identifier, PCI::RegisterOffset field)
|
|
{
|
|
VERIFY(identifier.operation_lock().is_locked());
|
|
return Access::the().read8_field(identifier, to_underlying(field));
|
|
}
|
|
u16 read16_locked(DeviceIdentifier const& identifier, PCI::RegisterOffset field)
|
|
{
|
|
VERIFY(identifier.operation_lock().is_locked());
|
|
return Access::the().read16_field(identifier, to_underlying(field));
|
|
}
|
|
u32 read32_locked(DeviceIdentifier const& identifier, PCI::RegisterOffset field)
|
|
{
|
|
VERIFY(identifier.operation_lock().is_locked());
|
|
return Access::the().read32_field(identifier, to_underlying(field));
|
|
}
|
|
|
|
ErrorOr<void> enumerate(Function<void(DeviceIdentifier const&)> callback)
|
|
{
|
|
return Access::the().fast_enumerate(callback);
|
|
}
|
|
|
|
HardwareID get_hardware_id(DeviceIdentifier const& identifier)
|
|
{
|
|
SpinlockLocker locker(identifier.operation_lock());
|
|
return { read16_locked(identifier, PCI::RegisterOffset::VENDOR_ID), read16_locked(identifier, PCI::RegisterOffset::DEVICE_ID) };
|
|
}
|
|
|
|
void enable_io_space(DeviceIdentifier const& identifier)
|
|
{
|
|
SpinlockLocker locker(identifier.operation_lock());
|
|
write16_locked(identifier, PCI::RegisterOffset::COMMAND, read16_locked(identifier, PCI::RegisterOffset::COMMAND) | (1 << 0));
|
|
}
|
|
void disable_io_space(DeviceIdentifier const& identifier)
|
|
{
|
|
SpinlockLocker locker(identifier.operation_lock());
|
|
write16_locked(identifier, PCI::RegisterOffset::COMMAND, read16_locked(identifier, PCI::RegisterOffset::COMMAND) & ~(1 << 0));
|
|
}
|
|
|
|
void enable_memory_space(DeviceIdentifier const& identifier)
|
|
{
|
|
SpinlockLocker locker(identifier.operation_lock());
|
|
write16_locked(identifier, PCI::RegisterOffset::COMMAND, read16_locked(identifier, PCI::RegisterOffset::COMMAND) | (1 << 1));
|
|
}
|
|
void disable_memory_space(DeviceIdentifier const& identifier)
|
|
{
|
|
SpinlockLocker locker(identifier.operation_lock());
|
|
write16_locked(identifier, PCI::RegisterOffset::COMMAND, read16_locked(identifier, PCI::RegisterOffset::COMMAND) & ~(1 << 1));
|
|
}
|
|
|
|
bool is_io_space_enabled(DeviceIdentifier const& identifier)
|
|
{
|
|
SpinlockLocker locker(identifier.operation_lock());
|
|
return (read16_locked(identifier, PCI::RegisterOffset::COMMAND) & 1) != 0;
|
|
}
|
|
|
|
void enable_interrupt_line(DeviceIdentifier const& identifier)
|
|
{
|
|
SpinlockLocker locker(identifier.operation_lock());
|
|
write16_locked(identifier, PCI::RegisterOffset::COMMAND, read16_locked(identifier, PCI::RegisterOffset::COMMAND) & ~(1 << 10));
|
|
}
|
|
|
|
void disable_interrupt_line(DeviceIdentifier const& identifier)
|
|
{
|
|
SpinlockLocker locker(identifier.operation_lock());
|
|
write16_locked(identifier, PCI::RegisterOffset::COMMAND, read16_locked(identifier, PCI::RegisterOffset::COMMAND) | 1 << 10);
|
|
}
|
|
|
|
u32 get_BAR0(DeviceIdentifier const& identifier)
|
|
{
|
|
SpinlockLocker locker(identifier.operation_lock());
|
|
return read32_locked(identifier, PCI::RegisterOffset::BAR0);
|
|
}
|
|
|
|
u32 get_BAR1(DeviceIdentifier const& identifier)
|
|
{
|
|
SpinlockLocker locker(identifier.operation_lock());
|
|
return read32_locked(identifier, PCI::RegisterOffset::BAR1);
|
|
}
|
|
|
|
u32 get_BAR2(DeviceIdentifier const& identifier)
|
|
{
|
|
SpinlockLocker locker(identifier.operation_lock());
|
|
return read32_locked(identifier, PCI::RegisterOffset::BAR2);
|
|
}
|
|
|
|
u32 get_BAR3(DeviceIdentifier const& identifier)
|
|
{
|
|
SpinlockLocker locker(identifier.operation_lock());
|
|
return read32_locked(identifier, PCI::RegisterOffset::BAR3);
|
|
}
|
|
|
|
u32 get_BAR4(DeviceIdentifier const& identifier)
|
|
{
|
|
SpinlockLocker locker(identifier.operation_lock());
|
|
return read32_locked(identifier, PCI::RegisterOffset::BAR4);
|
|
}
|
|
|
|
u32 get_BAR5(DeviceIdentifier const& identifier)
|
|
{
|
|
SpinlockLocker locker(identifier.operation_lock());
|
|
return read32_locked(identifier, PCI::RegisterOffset::BAR5);
|
|
}
|
|
|
|
u32 get_BAR(DeviceIdentifier const& identifier, HeaderType0BaseRegister pci_bar)
|
|
{
|
|
VERIFY(to_underlying(pci_bar) <= 5);
|
|
switch (to_underlying(pci_bar)) {
|
|
case 0:
|
|
return get_BAR0(identifier);
|
|
case 1:
|
|
return get_BAR1(identifier);
|
|
case 2:
|
|
return get_BAR2(identifier);
|
|
case 3:
|
|
return get_BAR3(identifier);
|
|
case 4:
|
|
return get_BAR4(identifier);
|
|
case 5:
|
|
return get_BAR5(identifier);
|
|
default:
|
|
VERIFY_NOT_REACHED();
|
|
}
|
|
}
|
|
|
|
BARSpaceType get_BAR_space_type(u32 pci_bar_value)
|
|
{
|
|
// Note: For IO space, bit 0 is set to 1.
|
|
if (pci_bar_value & (1 << 0))
|
|
return BARSpaceType::IOSpace;
|
|
auto memory_space_type = (pci_bar_value >> 1) & 0b11;
|
|
switch (memory_space_type) {
|
|
case 0:
|
|
return BARSpaceType::Memory32BitSpace;
|
|
case 1:
|
|
return BARSpaceType::Memory16BitSpace;
|
|
case 2:
|
|
return BARSpaceType::Memory64BitSpace;
|
|
default:
|
|
VERIFY_NOT_REACHED();
|
|
}
|
|
}
|
|
|
|
void enable_bus_mastering(DeviceIdentifier const& identifier)
|
|
{
|
|
SpinlockLocker locker(identifier.operation_lock());
|
|
auto value = read16_locked(identifier, PCI::RegisterOffset::COMMAND);
|
|
value |= (1 << 2);
|
|
value |= (1 << 0);
|
|
write16_locked(identifier, PCI::RegisterOffset::COMMAND, value);
|
|
}
|
|
|
|
void disable_bus_mastering(DeviceIdentifier const& identifier)
|
|
{
|
|
SpinlockLocker locker(identifier.operation_lock());
|
|
auto value = read16_locked(identifier, PCI::RegisterOffset::COMMAND);
|
|
value &= ~(1 << 2);
|
|
value |= (1 << 0);
|
|
write16_locked(identifier, PCI::RegisterOffset::COMMAND, value);
|
|
}
|
|
|
|
static void write8_offsetted(DeviceIdentifier const& identifier, u32 field, u8 value)
|
|
{
|
|
VERIFY(identifier.operation_lock().is_locked());
|
|
Access::the().write8_field(identifier, field, value);
|
|
}
|
|
static void write16_offsetted(DeviceIdentifier const& identifier, u32 field, u16 value)
|
|
{
|
|
VERIFY(identifier.operation_lock().is_locked());
|
|
Access::the().write16_field(identifier, field, value);
|
|
}
|
|
static void write32_offsetted(DeviceIdentifier const& identifier, u32 field, u32 value)
|
|
{
|
|
VERIFY(identifier.operation_lock().is_locked());
|
|
Access::the().write32_field(identifier, field, value);
|
|
}
|
|
static u8 read8_offsetted(DeviceIdentifier const& identifier, u32 field)
|
|
{
|
|
VERIFY(identifier.operation_lock().is_locked());
|
|
return Access::the().read8_field(identifier, field);
|
|
}
|
|
static u16 read16_offsetted(DeviceIdentifier const& identifier, u32 field)
|
|
{
|
|
VERIFY(identifier.operation_lock().is_locked());
|
|
return Access::the().read16_field(identifier, field);
|
|
}
|
|
static u32 read32_offsetted(DeviceIdentifier const& identifier, u32 field)
|
|
{
|
|
VERIFY(identifier.operation_lock().is_locked());
|
|
return Access::the().read32_field(identifier, field);
|
|
}
|
|
|
|
size_t get_BAR_space_size(DeviceIdentifier const& identifier, HeaderType0BaseRegister pci_bar)
|
|
{
|
|
SpinlockLocker locker(identifier.operation_lock());
|
|
// See PCI Spec 2.3, Page 222
|
|
VERIFY(to_underlying(pci_bar) < 6);
|
|
u8 field = to_underlying(PCI::RegisterOffset::BAR0) + (to_underlying(pci_bar) << 2);
|
|
u32 bar_reserved = read32_offsetted(identifier, field);
|
|
write32_offsetted(identifier, field, 0xFFFFFFFF);
|
|
u32 space_size = read32_offsetted(identifier, field);
|
|
write32_offsetted(identifier, field, bar_reserved);
|
|
space_size &= 0xfffffff0;
|
|
space_size = (~space_size) + 1;
|
|
return space_size;
|
|
}
|
|
|
|
void raw_access(DeviceIdentifier const& identifier, u32 field, size_t access_size, u32 value)
|
|
{
|
|
SpinlockLocker locker(identifier.operation_lock());
|
|
VERIFY(access_size != 0);
|
|
if (access_size == 1) {
|
|
write8_offsetted(identifier, field, value);
|
|
return;
|
|
}
|
|
if (access_size == 2) {
|
|
write16_offsetted(identifier, field, value);
|
|
return;
|
|
}
|
|
if (access_size == 4) {
|
|
write32_offsetted(identifier, field, value);
|
|
return;
|
|
}
|
|
VERIFY_NOT_REACHED();
|
|
}
|
|
|
|
u8 Capability::read8(size_t offset) const
|
|
{
|
|
auto& identifier = get_device_identifier(m_address);
|
|
SpinlockLocker locker(identifier.operation_lock());
|
|
return read8_offsetted(identifier, m_ptr + offset);
|
|
}
|
|
|
|
u16 Capability::read16(size_t offset) const
|
|
{
|
|
auto& identifier = get_device_identifier(m_address);
|
|
SpinlockLocker locker(identifier.operation_lock());
|
|
return read16_offsetted(identifier, m_ptr + offset);
|
|
}
|
|
|
|
u32 Capability::read32(size_t offset) const
|
|
{
|
|
auto& identifier = get_device_identifier(m_address);
|
|
SpinlockLocker locker(identifier.operation_lock());
|
|
return read32_offsetted(identifier, m_ptr + offset);
|
|
}
|
|
|
|
DeviceIdentifier const& get_device_identifier(Address address)
|
|
{
|
|
return Access::the().get_device_identifier(address);
|
|
}
|
|
|
|
}
|