mirror of
https://github.com/RGBCube/serenity
synced 2025-05-22 16:45:08 +00:00
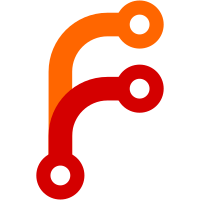
This implementation is basically a copy-paste of the SECP256r1 implementation with all "256" replaced with "384". In the future it might be nice to make this generic, instead of having two almost identical copies of code.
26 lines
782 B
C++
26 lines
782 B
C++
/*
|
|
* Copyright (c) 2023, Michiel Visser <opensource@webmichiel.nl>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <AK/ByteBuffer.h>
|
|
#include <AK/UFixedBigInt.h>
|
|
#include <LibCrypto/Curves/EllipticCurve.h>
|
|
|
|
namespace Crypto::Curves {
|
|
|
|
class SECP384r1 : public EllipticCurve {
|
|
public:
|
|
size_t key_size() override { return 1 + 2 * 48; }
|
|
ErrorOr<ByteBuffer> generate_private_key() override;
|
|
ErrorOr<ByteBuffer> generate_public_key(ReadonlyBytes a) override;
|
|
ErrorOr<ByteBuffer> compute_coordinate(ReadonlyBytes scalar_bytes, ReadonlyBytes point_bytes) override;
|
|
ErrorOr<ByteBuffer> derive_premaster_key(ReadonlyBytes shared_point) override;
|
|
|
|
ErrorOr<bool> verify(ReadonlyBytes hash, ReadonlyBytes pubkey, ReadonlyBytes signature);
|
|
};
|
|
|
|
}
|