mirror of
https://github.com/RGBCube/serenity
synced 2025-07-16 13:57:35 +00:00
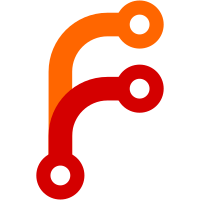
At any one given time, there can be an abitrary number of USB drivers in the system. The way driver mapping works (i.e, a device is inserted, and a potentially matching driver is probed) requires us to have instantiated driver objects _before_ a device is inserted. This leaves us with a slight "chicken and egg" problem. We cannot call the probe function before the driver is initialised, but we need to know _what_ driver to initialise. This section is designed to store pointers to functions that are called during the last stage of the early `_init` sequence in the Kernel. The accompanying macro in `USBDriver` emits a symbol, based on the driver name, into this table that is then automatically called. This way, we enforce a "common" driver model; driver developers are not only required to write their driver and inherit from `USB::Driver`, but are also required to have a free floating init function that registers their driver with the USB Core.
39 lines
884 B
C++
39 lines
884 B
C++
/*
|
|
* Copyright (c) 2023, Jesse Buhagiar <jesse.buhagiar@serenityos.org>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <AK/AtomicRefCounted.h>
|
|
#include <AK/Error.h>
|
|
#include <AK/NonnullRefPtr.h>
|
|
|
|
namespace Kernel::USB {
|
|
|
|
using DriverInitFunction = void (*)();
|
|
#define USB_DEVICE_DRIVER(driver_name) \
|
|
DriverInitFunction driver_init_function_ptr_##driver_name __attribute__((section(".driver_init"), used)) = &driver_name::init
|
|
|
|
class Device;
|
|
struct USBDeviceDescriptor;
|
|
class USBInterface;
|
|
|
|
class Driver : public AtomicRefCounted<Driver> {
|
|
public:
|
|
Driver(StringView name)
|
|
: m_driver_name(name)
|
|
{
|
|
}
|
|
|
|
virtual ~Driver() = default;
|
|
|
|
virtual ErrorOr<void> probe(USB::Device&) = 0;
|
|
virtual void detach(USB::Device&) = 0;
|
|
StringView name() const { return m_driver_name; }
|
|
|
|
protected:
|
|
StringView const m_driver_name;
|
|
};
|
|
}
|