mirror of
https://github.com/RGBCube/serenity
synced 2025-05-14 09:24:57 +00:00
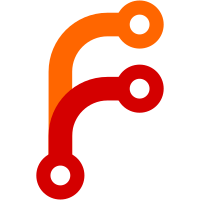
POSIX just says that mbstate_t should be an "object type other than an array type" that can hold the conversion state for converting between (multi-byte) characters and wide characters. Since no other information regarding the contents is given, this apparently means that we should add whatever we need once we start implementing that conversion.
38 lines
929 B
C
38 lines
929 B
C
/*
|
|
* Copyright (c) 2018-2020, Andreas Kling <kling@serenityos.org>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <stddef.h>
|
|
#include <sys/cdefs.h>
|
|
|
|
__BEGIN_DECLS
|
|
|
|
#ifndef WEOF
|
|
# define WEOF (0xffffffffu)
|
|
#endif
|
|
|
|
typedef __WINT_TYPE__ wint_t;
|
|
typedef unsigned long int wctype_t;
|
|
|
|
typedef struct {
|
|
} mbstate_t;
|
|
|
|
size_t wcslen(const wchar_t*);
|
|
wchar_t* wcscpy(wchar_t*, const wchar_t*);
|
|
wchar_t* wcsncpy(wchar_t*, const wchar_t*, size_t);
|
|
int wcscmp(const wchar_t*, const wchar_t*);
|
|
int wcsncmp(const wchar_t*, const wchar_t*, size_t);
|
|
wchar_t* wcschr(const wchar_t*, int);
|
|
const wchar_t* wcsrchr(const wchar_t*, wchar_t);
|
|
wchar_t* wcscat(wchar_t*, const wchar_t*);
|
|
wchar_t* wcsncat(wchar_t*, const wchar_t*, size_t);
|
|
wchar_t* wcstok(wchar_t*, const wchar_t*, wchar_t**);
|
|
long wcstol(const wchar_t*, wchar_t**, int);
|
|
long long wcstoll(const wchar_t*, wchar_t**, int);
|
|
wint_t btowc(int c);
|
|
|
|
__END_DECLS
|