mirror of
https://github.com/RGBCube/serenity
synced 2025-07-05 03:37:35 +00:00
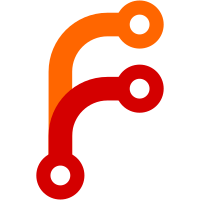
In the near future, we will be able to figure out connections between storage devices and their partitions, so there's no need to hardcode 16 partitions per storage device - each storage device should be able to have "infinite" count of partitions in it, and we should be able to use and figure out about them.
62 lines
1.7 KiB
C++
62 lines
1.7 KiB
C++
/*
|
|
* Copyright (c) 2020-2022, Liav A. <liavalb@hotmail.co.il>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <AK/IntrusiveList.h>
|
|
#include <AK/NonnullRefPtr.h>
|
|
#include <AK/NonnullRefPtrVector.h>
|
|
#include <AK/Types.h>
|
|
#include <Kernel/FileSystem/FileSystem.h>
|
|
#include <Kernel/Storage/DiskPartition.h>
|
|
#include <Kernel/Storage/StorageController.h>
|
|
#include <Kernel/Storage/StorageDevice.h>
|
|
#include <LibPartition/PartitionTable.h>
|
|
|
|
namespace Kernel {
|
|
|
|
class StorageManagement {
|
|
|
|
public:
|
|
StorageManagement();
|
|
static bool initialized();
|
|
void initialize(StringView boot_argument, bool force_pio, bool nvme_poll);
|
|
static StorageManagement& the();
|
|
|
|
NonnullRefPtr<FileSystem> root_filesystem() const;
|
|
|
|
static MajorNumber storage_type_major_number();
|
|
static MinorNumber generate_storage_minor_number();
|
|
|
|
static MinorNumber generate_partition_minor_number();
|
|
|
|
static u32 generate_controller_id();
|
|
|
|
void remove_device(StorageDevice&);
|
|
|
|
private:
|
|
bool boot_argument_contains_partition_uuid();
|
|
|
|
void enumerate_pci_controllers(bool force_pio, bool nvme_poll);
|
|
void enumerate_storage_devices();
|
|
void enumerate_disk_partitions();
|
|
|
|
void determine_boot_device();
|
|
void determine_boot_device_with_partition_uuid();
|
|
|
|
void dump_storage_devices_and_partitions() const;
|
|
|
|
ErrorOr<NonnullOwnPtr<Partition::PartitionTable>> try_to_initialize_partition_table(StorageDevice const&) const;
|
|
|
|
RefPtr<BlockDevice> boot_block_device() const;
|
|
|
|
StringView m_boot_argument;
|
|
WeakPtr<BlockDevice> m_boot_block_device;
|
|
NonnullRefPtrVector<StorageController> m_controllers;
|
|
IntrusiveList<&StorageDevice::m_list_node> m_storage_devices;
|
|
};
|
|
|
|
}
|