mirror of
https://github.com/RGBCube/serenity
synced 2025-05-14 09:34:59 +00:00
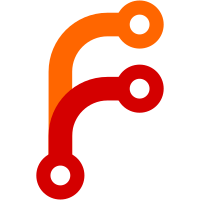
Before this patch, if two or more notifications were created after one another, they would overlap. This was caused by the previously lowest notification's m_original_rect being used to calculate the position for each new notification instead of the notification's actual rect, which can be different. This patch makes notifications use each others' rect() method instead, which makes them appear in the correct position. With that, m_original_rect has no use anymore, so this patch removes it.
46 lines
1.1 KiB
C++
46 lines
1.1 KiB
C++
/*
|
|
* Copyright (c) 2020, Andreas Kling <kling@serenityos.org>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <LibGUI/ImageWidget.h>
|
|
#include <LibGUI/Window.h>
|
|
|
|
namespace NotificationServer {
|
|
|
|
class NotificationWindow final : public GUI::Window {
|
|
C_OBJECT(NotificationWindow);
|
|
|
|
public:
|
|
virtual ~NotificationWindow() override = default;
|
|
|
|
void set_text(String const&);
|
|
void set_title(String const&);
|
|
void set_image(Gfx::ShareableBitmap const&);
|
|
|
|
static RefPtr<NotificationWindow> get_window_by_id(i32 id);
|
|
|
|
protected:
|
|
virtual void enter_event(Core::Event&) override;
|
|
virtual void leave_event(Core::Event&) override;
|
|
|
|
private:
|
|
NotificationWindow(i32 client_id, String const& text, String const& title, Gfx::ShareableBitmap const&);
|
|
|
|
virtual void screen_rects_change_event(GUI::ScreenRectsChangeEvent&) override;
|
|
|
|
void resize_to_fit_text();
|
|
void set_height(int);
|
|
|
|
i32 m_id;
|
|
|
|
GUI::Label* m_text_label;
|
|
GUI::Label* m_title_label;
|
|
GUI::ImageWidget* m_image;
|
|
bool m_hovering { false };
|
|
};
|
|
|
|
}
|