mirror of
https://github.com/RGBCube/serenity
synced 2025-05-14 08:34:57 +00:00
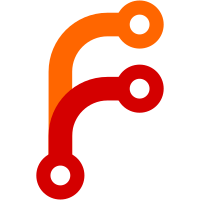
This device is a block device that allows a user to effectively treat an Inode as a block device. The static construction method is given an OpenFileDescription reference but validates that: - The description has a valid custody (so it's not some arbitrary file). Failing this requirement will yield EINVAL. - The description custody points to an Inode which is a regular file, as we only support (seekable) regular files. Failing this requirement will yield ENOTSUP. LoopDevice can be used to mount a regular file on the filesystem like other supported types of (physical) block devices.
62 lines
2 KiB
C++
62 lines
2 KiB
C++
/*
|
|
* Copyright (c) 2024, Liav A. <liavalb@hotmail.co.il>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <AK/Badge.h>
|
|
#include <AK/IntrusiveList.h>
|
|
#include <Kernel/Devices/BlockDevice.h>
|
|
#include <Kernel/FileSystem/Inode.h>
|
|
#include <Kernel/Forward.h>
|
|
#include <Kernel/Library/ListedRefCounted.h>
|
|
|
|
namespace Kernel {
|
|
|
|
class LoopDevice final : public BlockDevice {
|
|
friend class DeviceManagement;
|
|
|
|
public:
|
|
virtual bool unref() const override;
|
|
virtual ~LoopDevice() = default;
|
|
|
|
void remove(Badge<DeviceControlDevice>);
|
|
static ErrorOr<NonnullRefPtr<LoopDevice>> create_with_file_description(OpenFileDescription&);
|
|
|
|
virtual StringView class_name() const override { return "LoopDevice"sv; }
|
|
|
|
virtual ErrorOr<size_t> read(OpenFileDescription&, u64, UserOrKernelBuffer&, size_t) override;
|
|
virtual ErrorOr<size_t> write(OpenFileDescription&, u64, UserOrKernelBuffer const&, size_t) override;
|
|
virtual bool can_read(OpenFileDescription const&, u64) const override;
|
|
virtual bool can_write(OpenFileDescription const&, u64) const override;
|
|
virtual ErrorOr<void> ioctl(OpenFileDescription&, unsigned request, Userspace<void*> arg) override;
|
|
|
|
virtual bool is_loop_device() const override { return true; }
|
|
|
|
unsigned index() const { return m_index; }
|
|
|
|
Inode const& inode() const { return m_backing_custody->inode(); }
|
|
Inode& inode() { return m_backing_custody->inode(); }
|
|
|
|
Custody const& custody() const { return *m_backing_custody; }
|
|
Custody& custody() { return *m_backing_custody; }
|
|
|
|
private:
|
|
virtual void start_request(AsyncBlockDeviceRequest&) override;
|
|
|
|
LoopDevice(NonnullRefPtr<Custody>, unsigned index);
|
|
|
|
NonnullRefPtr<Custody> const m_backing_custody;
|
|
unsigned const m_index { 0 };
|
|
|
|
mutable IntrusiveListNode<LoopDevice, NonnullRefPtr<LoopDevice>> m_list_node;
|
|
|
|
public:
|
|
using List = IntrusiveList<&LoopDevice::m_list_node>;
|
|
|
|
static SpinlockProtected<LoopDevice::List, LockRank::None>& all_instances();
|
|
};
|
|
|
|
}
|