mirror of
https://github.com/RGBCube/serenity
synced 2025-05-23 17:45:07 +00:00
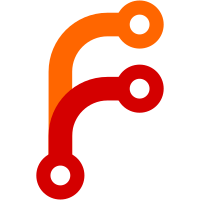
Note that this does nothing to "fix" how element references are created. We continue to return the element ID because, otherwise, all other element WebDriver endpoints would break. On the bright side, we avoid several IPC round trips now that we perform the entire 'find' operation in the WebContent process; and we are able to work directly on DOM nodes.
54 lines
2.3 KiB
C++
54 lines
2.3 KiB
C++
/*
|
|
* Copyright (c) 2022, Florent Castelli <florent.castelli@gmail.com>
|
|
* Copyright (c) 2022, Linus Groh <linusg@serenityos.org>
|
|
* Copyright (c) 2022, Tim Flynn <trflynn89@serenityos.org>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <LibIPC/ConnectionToServer.h>
|
|
#include <LibWeb/Forward.h>
|
|
#include <LibWeb/WebDriver/ElementLocationStrategies.h>
|
|
#include <LibWeb/WebDriver/Response.h>
|
|
#include <WebContent/Forward.h>
|
|
#include <WebContent/WebDriverClientEndpoint.h>
|
|
#include <WebContent/WebDriverServerEndpoint.h>
|
|
|
|
namespace WebContent {
|
|
|
|
class WebDriverConnection final
|
|
: public IPC::ConnectionToServer<WebDriverClientEndpoint, WebDriverServerEndpoint> {
|
|
C_OBJECT_ABSTRACT(WebDriverConnection)
|
|
|
|
public:
|
|
static ErrorOr<NonnullRefPtr<WebDriverConnection>> connect(ConnectionFromClient& web_content_client, PageHost& page_host, String const& webdriver_ipc_path);
|
|
virtual ~WebDriverConnection() = default;
|
|
|
|
private:
|
|
WebDriverConnection(NonnullOwnPtr<Core::Stream::LocalSocket> socket, ConnectionFromClient& web_content_client, PageHost& page_host);
|
|
|
|
virtual void die() override { }
|
|
|
|
virtual void close_session() override;
|
|
virtual void set_is_webdriver_active(bool) override;
|
|
virtual Messages::WebDriverClient::NavigateToResponse navigate_to(JsonValue const& payload) override;
|
|
virtual Messages::WebDriverClient::GetCurrentUrlResponse get_current_url() override;
|
|
virtual Messages::WebDriverClient::GetWindowRectResponse get_window_rect() override;
|
|
virtual Messages::WebDriverClient::SetWindowRectResponse set_window_rect(JsonValue const& payload) override;
|
|
virtual Messages::WebDriverClient::MaximizeWindowResponse maximize_window() override;
|
|
virtual Messages::WebDriverClient::MinimizeWindowResponse minimize_window() override;
|
|
virtual Messages::WebDriverClient::FindElementResponse find_element(JsonValue const& payload) override;
|
|
|
|
ErrorOr<void, Web::WebDriver::Error> ensure_open_top_level_browsing_context();
|
|
void restore_the_window();
|
|
Gfx::IntRect maximize_the_window();
|
|
Gfx::IntRect iconify_the_window();
|
|
ErrorOr<JsonArray, Web::WebDriver::Error> find(Web::DOM::ParentNode& start_node, Web::WebDriver::LocationStrategy using_, StringView value);
|
|
|
|
ConnectionFromClient& m_web_content_client;
|
|
PageHost& m_page_host;
|
|
};
|
|
|
|
}
|