mirror of
https://github.com/RGBCube/serenity
synced 2025-05-14 07:54:58 +00:00
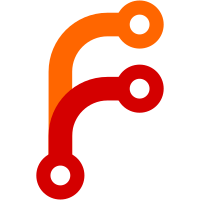
This makes construction of Utf16String fallible in OOM conditions. The immediate impact is that PrimitiveString must then be fallible as well, as it may either transcode UTF-8 to UTF-16, or create a UTF-16 string from ropes. There are a couple of places where it is very non-trivial to propagate the error further. A FIXME has been added to those locations.
45 lines
1.6 KiB
C++
45 lines
1.6 KiB
C++
/*
|
|
* Copyright (c) 2020-2021, Andreas Kling <kling@serenityos.org>
|
|
* Copyright (c) 2020-2021, Linus Groh <linusg@serenityos.org>
|
|
* Copyright (c) 2020-2022, Idan Horowitz <idan.horowitz@serenityos.org>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <LibJS/Runtime/PrimitiveString.h>
|
|
#include <LibJS/Runtime/Value.h>
|
|
|
|
namespace JS {
|
|
struct ValueTraits : public Traits<Value> {
|
|
static unsigned hash(Value value)
|
|
{
|
|
VERIFY(!value.is_empty());
|
|
if (value.is_string()) {
|
|
// FIXME: Propagate this error.
|
|
return value.as_string().deprecated_string().release_value().hash();
|
|
}
|
|
|
|
if (value.is_bigint())
|
|
return value.as_bigint().big_integer().hash();
|
|
|
|
if (value.is_negative_zero())
|
|
value = Value(0);
|
|
// In the IEEE 754 standard a NaN value is encoded as any value from 0x7ff0000000000001 to 0x7fffffffffffffff,
|
|
// with the least significant bits (referred to as the 'payload') carrying some kind of diagnostic information
|
|
// indicating the source of the NaN. Since ECMA262 does not differentiate between different kinds of NaN values,
|
|
// Sets and Maps must not differentiate between them either.
|
|
// This is achieved by replacing any NaN value by a canonical qNaN.
|
|
else if (value.is_nan())
|
|
value = js_nan();
|
|
|
|
return u64_hash(value.encoded()); // FIXME: Is this the best way to hash pointers, doubles & ints?
|
|
}
|
|
static bool equals(const Value a, const Value b)
|
|
{
|
|
return same_value_zero(a, b);
|
|
}
|
|
};
|
|
|
|
}
|