mirror of
https://github.com/RGBCube/serenity
synced 2025-05-22 16:45:08 +00:00
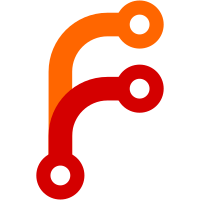
Most of the models were just calling did_update anyway, which is pointless since it can be unified to the base Model class. Instead, code calling update() will now call invalidate(), which functions identically and is more obvious in what it does. Additionally, a default implementation is provided, which removes the need to add empty implementations of update() for each model subclass. Co-Authored-By: Ali Mohammad Pur <ali.mpfard@gmail.com>
58 lines
1.5 KiB
C++
58 lines
1.5 KiB
C++
/*
|
|
* Copyright (c) 2021, Cesar Torres <shortanemoia@protonmail.com>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include "M3UParser.h"
|
|
#include <LibGUI/Model.h>
|
|
#include <LibGUI/TableView.h>
|
|
#include <LibGUI/Variant.h>
|
|
#include <LibGUI/Widget.h>
|
|
|
|
enum class PlaylistModelCustomRole {
|
|
_DONOTUSE = (int)GUI::ModelRole::Custom,
|
|
FilePath
|
|
};
|
|
|
|
class PlaylistModel : public GUI::Model {
|
|
public:
|
|
~PlaylistModel() override = default;
|
|
|
|
int row_count(const GUI::ModelIndex&) const override { return m_playlist_items.size(); }
|
|
int column_count(const GUI::ModelIndex&) const override { return 6; }
|
|
GUI::Variant data(const GUI::ModelIndex&, GUI::ModelRole) const override;
|
|
String column_name(int column) const override;
|
|
Vector<M3UEntry>& items() { return m_playlist_items; }
|
|
|
|
private:
|
|
Vector<M3UEntry> m_playlist_items;
|
|
|
|
static String format_filesize(u64 size_in_bytes);
|
|
static String format_duration(u32 duration_in_seconds);
|
|
};
|
|
|
|
class PlaylistTableView : public GUI::TableView {
|
|
C_OBJECT(PlaylistTableView)
|
|
public:
|
|
void doubleclick_event(GUI::MouseEvent& event) override;
|
|
|
|
Function<void(const Gfx::Point<int>&)> on_doubleclick;
|
|
};
|
|
|
|
class PlaylistWidget : public GUI::Widget {
|
|
C_OBJECT(PlaylistWidget)
|
|
public:
|
|
PlaylistWidget();
|
|
void set_data_model(RefPtr<PlaylistModel> model)
|
|
{
|
|
m_table_view->set_model(model);
|
|
m_table_view->update();
|
|
}
|
|
|
|
protected:
|
|
private:
|
|
RefPtr<PlaylistTableView> m_table_view;
|
|
};
|