mirror of
https://github.com/RGBCube/serenity
synced 2025-07-12 16:17:36 +00:00
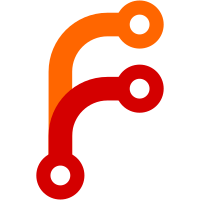
This patch makes it possible to call Node::invalidate_style() and have that node and all of its ancestors recompute their style. We then figure out if the new style is visually different from the old style, and if so do a paint invalidation with set_needs_display(). Note that the "are they visually different" code is very incomplete! Use this to make hover effects a lot more efficient. They no longer cause a full relayout+repaint, but only a style invalidation. Style invalidations are still quite heavy though, and there's a lot of room for improvement there. :^)
70 lines
1.9 KiB
C++
70 lines
1.9 KiB
C++
#pragma once
|
|
|
|
#include <AK/String.h>
|
|
#include <LibHTML/DOM/ParentNode.h>
|
|
#include <LibHTML/Layout/LayoutNode.h>
|
|
|
|
class LayoutNodeWithStyle;
|
|
|
|
class Attribute {
|
|
public:
|
|
Attribute(const String& name, const String& value)
|
|
: m_name(name)
|
|
, m_value(value)
|
|
{
|
|
}
|
|
|
|
const String& name() const { return m_name; }
|
|
const String& value() const { return m_value; }
|
|
|
|
void set_value(const String& value) { m_value = value; }
|
|
|
|
private:
|
|
String m_name;
|
|
String m_value;
|
|
};
|
|
|
|
class Element : public ParentNode {
|
|
public:
|
|
Element(Document&, const String& tag_name);
|
|
virtual ~Element() override;
|
|
|
|
virtual String tag_name() const final { return m_tag_name; }
|
|
|
|
String attribute(const String& name) const;
|
|
void set_attribute(const String& name, const String& value);
|
|
|
|
void set_attributes(Vector<Attribute>&&);
|
|
|
|
template<typename Callback>
|
|
void for_each_attribute(Callback callback) const
|
|
{
|
|
for (auto& attribute : m_attributes)
|
|
callback(attribute.name(), attribute.value());
|
|
}
|
|
|
|
bool has_class(const StringView&) const;
|
|
|
|
virtual void apply_presentational_hints(StyleProperties&) const {}
|
|
virtual void parse_attribute(const String& name, const String& value);
|
|
|
|
void recompute_style();
|
|
|
|
LayoutNodeWithStyle* layout_node() { return static_cast<LayoutNodeWithStyle*>(Node::layout_node()); }
|
|
const LayoutNodeWithStyle* layout_node() const { return static_cast<const LayoutNodeWithStyle*>(Node::layout_node()); }
|
|
|
|
private:
|
|
RefPtr<LayoutNode> create_layout_node(const StyleResolver&, const StyleProperties* parent_style) const override;
|
|
|
|
Attribute* find_attribute(const String& name);
|
|
const Attribute* find_attribute(const String& name) const;
|
|
|
|
String m_tag_name;
|
|
Vector<Attribute> m_attributes;
|
|
};
|
|
|
|
template<>
|
|
inline bool is<Element>(const Node& node)
|
|
{
|
|
return node.is_element();
|
|
}
|