mirror of
https://github.com/RGBCube/serenity
synced 2025-07-05 21:47:36 +00:00
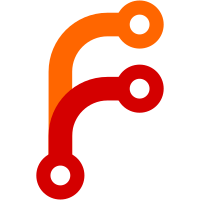
It might be the case that we are passing non-movable/non-copyable things through IPC. In this case, Clang will emit a warning as it can't generate the requested default move/copy ctor for the IPC message. To fix this, we use a `#pragma` to make the compiler silently ignore our request. The same was the case with the three-way comparison in `Screen`. Since we don't use the three-way comparison operator anywhere else in our codebase, we simply use the `==` operator instead.
60 lines
1.5 KiB
C++
60 lines
1.5 KiB
C++
/*
|
|
* Copyright (c) 2020, the SerenityOS developers.
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <AK/String.h>
|
|
#include <AK/Vector.h>
|
|
#include <LibCore/ConfigFile.h>
|
|
#include <LibGfx/Rect.h>
|
|
#include <LibGfx/Size.h>
|
|
#include <LibIPC/Forward.h>
|
|
|
|
namespace WindowServer {
|
|
|
|
class ScreenLayout {
|
|
public:
|
|
struct Screen {
|
|
String device;
|
|
Gfx::IntPoint location;
|
|
Gfx::IntSize resolution;
|
|
int scale_factor;
|
|
|
|
Gfx::IntRect virtual_rect() const
|
|
{
|
|
return { location, { resolution.width() / scale_factor, resolution.height() / scale_factor } };
|
|
}
|
|
|
|
bool operator==(const Screen&) const = default;
|
|
};
|
|
|
|
Vector<Screen> screens;
|
|
unsigned main_screen_index { 0 };
|
|
|
|
bool is_valid(String* error_msg = nullptr) const;
|
|
void normalize();
|
|
bool load_config(const Core::ConfigFile& config_file, String* error_msg = nullptr);
|
|
bool save_config(Core::ConfigFile& config_file, bool sync = true) const;
|
|
bool try_auto_add_framebuffer(String const&);
|
|
|
|
// TODO: spaceship operator
|
|
bool operator!=(const ScreenLayout& other) const;
|
|
bool operator==(const ScreenLayout& other) const
|
|
{
|
|
return !(*this != other);
|
|
}
|
|
};
|
|
|
|
}
|
|
|
|
namespace IPC {
|
|
|
|
bool encode(Encoder&, const WindowServer::ScreenLayout::Screen&);
|
|
bool decode(Decoder&, WindowServer::ScreenLayout::Screen&);
|
|
bool encode(Encoder&, const WindowServer::ScreenLayout&);
|
|
bool decode(Decoder&, WindowServer::ScreenLayout&);
|
|
|
|
}
|