mirror of
https://github.com/RGBCube/serenity
synced 2025-05-27 23:25:08 +00:00
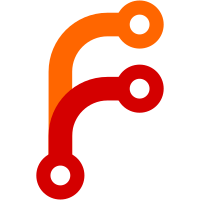
We'll be making a lot of trees here, so let's share code during bootstrap. Eventually some of these classes are gonna want custom trees but for now we can just fit them all into the same clothes.
29 lines
659 B
C++
29 lines
659 B
C++
#pragma once
|
|
|
|
#include <LibHTML/DOM/Node.h>
|
|
|
|
class ParentNode : public Node {
|
|
public:
|
|
template<typename F> void for_each_child(F) const;
|
|
template<typename F> void for_each_child(F);
|
|
|
|
protected:
|
|
explicit ParentNode(NodeType type)
|
|
: Node(type)
|
|
{
|
|
}
|
|
};
|
|
|
|
template<typename Callback>
|
|
inline void ParentNode::for_each_child(Callback callback) const
|
|
{
|
|
for (auto* node = first_child(); node; node = node->next_sibling())
|
|
callback(*node);
|
|
}
|
|
|
|
template<typename Callback>
|
|
inline void ParentNode::for_each_child(Callback callback)
|
|
{
|
|
for (auto* node = first_child(); node; node = node->next_sibling())
|
|
callback(*node);
|
|
}
|