mirror of
https://github.com/RGBCube/serenity
synced 2025-05-26 00:25:08 +00:00
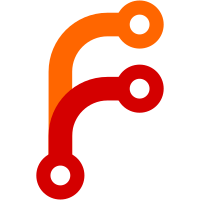
We'll be making a lot of trees here, so let's share code during bootstrap. Eventually some of these classes are gonna want custom trees but for now we can just fit them all into the same clothes.
52 lines
1.3 KiB
C++
52 lines
1.3 KiB
C++
#pragma once
|
|
|
|
#include <AK/NonnullRefPtr.h>
|
|
#include <AK/Vector.h>
|
|
#include <LibHTML/Layout/LayoutStyle.h>
|
|
#include <LibHTML/TreeNode.h>
|
|
#include <SharedGraphics/Rect.h>
|
|
|
|
class Node;
|
|
|
|
class LayoutNode : public TreeNode<LayoutNode> {
|
|
public:
|
|
virtual ~LayoutNode();
|
|
|
|
const Rect& rect() const { return m_rect; }
|
|
Rect& rect() { return m_rect; }
|
|
void set_rect(const Rect& rect) { m_rect = rect; }
|
|
|
|
LayoutStyle& style() { return m_style; }
|
|
const LayoutStyle& style() const { return m_style; }
|
|
|
|
bool is_anonymous() const { return !m_node; }
|
|
const Node* node() const { return m_node; }
|
|
|
|
template<typename Callback>
|
|
inline void for_each_child(Callback callback) const
|
|
{
|
|
for (auto* node = first_child(); node; node = node->next_sibling())
|
|
callback(*node);
|
|
}
|
|
|
|
template<typename Callback>
|
|
inline void for_each_child(Callback callback)
|
|
{
|
|
for (auto* node = first_child(); node; node = node->next_sibling())
|
|
callback(*node);
|
|
}
|
|
|
|
virtual const char* class_name() const { return "LayoutNode"; }
|
|
virtual bool is_text() const { return false; }
|
|
|
|
virtual void layout();
|
|
|
|
protected:
|
|
explicit LayoutNode(const Node*);
|
|
|
|
private:
|
|
const Node* m_node { nullptr };
|
|
|
|
LayoutStyle m_style;
|
|
Rect m_rect;
|
|
};
|