mirror of
https://github.com/RGBCube/serenity
synced 2025-07-12 18:37:36 +00:00
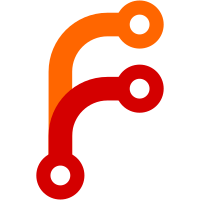
This fixes a small bug from 39b2eed3f6
: That commit tried to disable
filters for the very first object read, for the case covered in
Tests/LibPDF/password-is-sup.pdf.
However, it accidentally also disabled filters by default.
Most of the time, this isn't really a difference: We call
`set_filters_enabled(true);` very early in
`DocumentParser::initialize_linearization_dict()`, which explicitly
enables filters, and `initialize_linearization_dict()` is the very
first thing called in `DocumentParser::initialize()`.
But there's an early exit in `initialize_linearization_dict()`
for if there's nothing looking like an indirect object right
after the header, and in this case we used to not enable
filtering, and would hand compressed streams to the operand parser.
(And due to a 2nd bug, we'd even do this if the header line was
followed by an empty line.)
89 lines
2.4 KiB
C++
89 lines
2.4 KiB
C++
/*
|
|
* Copyright (c) 2021-2022, Matthew Olsson <mattco@serenityos.org>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <AK/SourceLocation.h>
|
|
#include <AK/WeakPtr.h>
|
|
#include <LibPDF/Object.h>
|
|
#include <LibPDF/Operator.h>
|
|
#include <LibPDF/Reader.h>
|
|
#include <LibPDF/XRefTable.h>
|
|
|
|
namespace PDF {
|
|
|
|
template<typename T, typename... Args>
|
|
static NonnullRefPtr<T> make_object(Args... args)
|
|
requires(IsBaseOf<Object, T>)
|
|
{
|
|
return adopt_ref(*new T(forward<Args>(args)...));
|
|
}
|
|
|
|
class Document;
|
|
|
|
class Parser {
|
|
public:
|
|
static PDFErrorOr<Vector<Operator>> parse_operators(Document*, ReadonlyBytes);
|
|
|
|
Parser(ReadonlyBytes);
|
|
Parser(Document*, ReadonlyBytes);
|
|
|
|
void set_document(WeakPtr<Document> const&);
|
|
|
|
DeprecatedString parse_comment();
|
|
|
|
void move_by(size_t count) { m_reader.move_by(count); }
|
|
void move_to(size_t offset) { m_reader.move_to(offset); }
|
|
|
|
enum class CanBeIndirectValue {
|
|
No,
|
|
Yes
|
|
};
|
|
|
|
PDFErrorOr<Value> parse_value(CanBeIndirectValue = CanBeIndirectValue::Yes);
|
|
PDFErrorOr<Value> parse_possible_indirect_value_or_ref();
|
|
PDFErrorOr<NonnullRefPtr<IndirectValue>> parse_indirect_value(u32 index, u32 generation);
|
|
PDFErrorOr<NonnullRefPtr<IndirectValue>> parse_indirect_value();
|
|
PDFErrorOr<Value> parse_number();
|
|
PDFErrorOr<NonnullRefPtr<NameObject>> parse_name();
|
|
NonnullRefPtr<StringObject> parse_string();
|
|
DeprecatedString parse_literal_string();
|
|
DeprecatedString parse_hex_string();
|
|
PDFErrorOr<NonnullRefPtr<ArrayObject>> parse_array();
|
|
PDFErrorOr<NonnullRefPtr<DictObject>> parse_dict();
|
|
PDFErrorOr<NonnullRefPtr<StreamObject>> parse_stream(NonnullRefPtr<DictObject> dict);
|
|
PDFErrorOr<Vector<Operator>> parse_operators();
|
|
|
|
void set_filters_enabled(bool enabled)
|
|
{
|
|
m_enable_filters = enabled;
|
|
}
|
|
|
|
void set_encryption_enabled(bool enabled)
|
|
{
|
|
m_enable_encryption = enabled;
|
|
}
|
|
|
|
void push_reference(Reference const& ref) { m_current_reference_stack.append(ref); }
|
|
void pop_reference() { m_current_reference_stack.take_last(); }
|
|
|
|
protected:
|
|
Error error(
|
|
DeprecatedString const& message
|
|
#ifdef PDF_DEBUG
|
|
,
|
|
SourceLocation loc = SourceLocation::current()
|
|
#endif
|
|
) const;
|
|
|
|
Reader m_reader;
|
|
WeakPtr<Document> m_document;
|
|
Vector<Reference> m_current_reference_stack;
|
|
bool m_enable_encryption { true };
|
|
bool m_enable_filters { true };
|
|
};
|
|
|
|
};
|