mirror of
https://github.com/RGBCube/serenity
synced 2025-07-05 21:57:35 +00:00
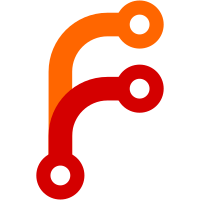
It is currently a bit messy to pass these options along from main() to where WebContent is actually launched. If a new flag were to be added, there are a couple dozen files that need to be updated to pass that flag along. With this change, the flag can just be added to the struct, set in main(), and handled in launch_web_content_process().
48 lines
1.5 KiB
Objective-C
48 lines
1.5 KiB
Objective-C
/*
|
|
* Copyright (c) 2023, Tim Flynn <trflynn89@serenityos.org>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <AK/Optional.h>
|
|
#include <AK/StringView.h>
|
|
#include <AK/URL.h>
|
|
#include <AK/Vector.h>
|
|
#include <Ladybird/Types.h>
|
|
#include <LibWeb/CSS/PreferredColorScheme.h>
|
|
#include <LibWeb/HTML/ActivateTab.h>
|
|
#include <LibWebView/CookieJar.h>
|
|
|
|
#import <System/Cocoa.h>
|
|
|
|
@class Tab;
|
|
@class TabController;
|
|
|
|
@interface ApplicationDelegate : NSObject <NSApplicationDelegate>
|
|
|
|
- (nullable instancetype)init:(Vector<URL>)initial_urls
|
|
newTabPageURL:(URL)new_tab_page_url
|
|
withCookieJar:(WebView::CookieJar)cookie_jar
|
|
webContentOptions:(Ladybird::WebContentOptions const&)web_content_options
|
|
webdriverContentIPCPath:(StringView)webdriver_content_ipc_path;
|
|
|
|
- (nonnull TabController*)createNewTab:(Optional<URL> const&)url
|
|
fromTab:(nullable Tab*)tab
|
|
activateTab:(Web::HTML::ActivateTab)activate_tab;
|
|
|
|
- (nonnull TabController*)createNewTab:(StringView)html
|
|
url:(URL const&)url
|
|
fromTab:(nullable Tab*)tab
|
|
activateTab:(Web::HTML::ActivateTab)activate_tab;
|
|
|
|
- (void)removeTab:(nonnull TabController*)controller;
|
|
|
|
- (WebView::CookieJar&)cookieJar;
|
|
- (Ladybird::WebContentOptions const&)webContentOptions;
|
|
- (Optional<StringView> const&)webdriverContentIPCPath;
|
|
- (Web::CSS::PreferredColorScheme)preferredColorScheme;
|
|
- (WebView::SearchEngine const&)searchEngine;
|
|
|
|
@end
|