mirror of
https://github.com/RGBCube/serenity
synced 2025-07-04 01:37:34 +00:00
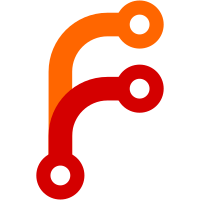
This commit un-deprecates DeprecatedString, and repurposes it as a byte string. As the null state has already been removed, there are no other particularly hairy blockers in repurposing this type as a byte string (what it _really_ is). This commit is auto-generated: $ xs=$(ack -l \bDeprecatedString\b\|deprecated_string AK Userland \ Meta Ports Ladybird Tests Kernel) $ perl -pie 's/\bDeprecatedString\b/ByteString/g; s/deprecated_string/byte_string/g' $xs $ clang-format --style=file -i \ $(git diff --name-only | grep \.cpp\|\.h) $ gn format $(git ls-files '*.gn' '*.gni')
128 lines
2.4 KiB
C++
128 lines
2.4 KiB
C++
/*
|
|
* Copyright (c) 2020-2022, the SerenityOS developers.
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <AK/ByteString.h>
|
|
#include <AK/Forward.h>
|
|
#include <AK/NonnullOwnPtr.h>
|
|
#include <AK/URL.h>
|
|
|
|
namespace Gemini {
|
|
|
|
class Line {
|
|
public:
|
|
Line(ByteString string)
|
|
: m_text(move(string))
|
|
{
|
|
}
|
|
|
|
virtual ~Line() = default;
|
|
|
|
virtual ByteString render_to_html() const = 0;
|
|
|
|
protected:
|
|
ByteString m_text;
|
|
};
|
|
|
|
class Document : public RefCounted<Document> {
|
|
public:
|
|
ByteString render_to_html() const;
|
|
|
|
static NonnullRefPtr<Document> parse(StringView source, const URL&);
|
|
|
|
const URL& url() const { return m_url; }
|
|
|
|
private:
|
|
explicit Document(const URL& url)
|
|
: m_url(url)
|
|
{
|
|
}
|
|
|
|
void read_lines(StringView);
|
|
|
|
Vector<NonnullOwnPtr<Line>> m_lines;
|
|
URL m_url;
|
|
bool m_inside_preformatted_block { false };
|
|
bool m_inside_unordered_list { false };
|
|
};
|
|
|
|
class Text : public Line {
|
|
public:
|
|
Text(ByteString line)
|
|
: Line(move(line))
|
|
{
|
|
}
|
|
virtual ~Text() override = default;
|
|
virtual ByteString render_to_html() const override;
|
|
};
|
|
|
|
class Link : public Line {
|
|
public:
|
|
Link(ByteString line, Document const&);
|
|
virtual ~Link() override = default;
|
|
virtual ByteString render_to_html() const override;
|
|
|
|
private:
|
|
URL m_url;
|
|
ByteString m_name;
|
|
};
|
|
|
|
class Preformatted : public Line {
|
|
public:
|
|
Preformatted(ByteString line)
|
|
: Line(move(line))
|
|
{
|
|
}
|
|
virtual ~Preformatted() override = default;
|
|
virtual ByteString render_to_html() const override;
|
|
};
|
|
|
|
class UnorderedList : public Line {
|
|
public:
|
|
UnorderedList(ByteString line)
|
|
: Line(move(line))
|
|
{
|
|
}
|
|
virtual ~UnorderedList() override = default;
|
|
virtual ByteString render_to_html() const override;
|
|
};
|
|
|
|
class Control : public Line {
|
|
public:
|
|
enum Kind {
|
|
UnorderedListStart,
|
|
UnorderedListEnd,
|
|
PreformattedStart,
|
|
PreformattedEnd,
|
|
};
|
|
Control(Kind kind)
|
|
: Line("")
|
|
, m_kind(kind)
|
|
{
|
|
}
|
|
virtual ~Control() override = default;
|
|
virtual ByteString render_to_html() const override;
|
|
|
|
private:
|
|
Kind m_kind;
|
|
};
|
|
|
|
class Heading : public Line {
|
|
public:
|
|
Heading(ByteString line, int level)
|
|
: Line(move(line))
|
|
, m_level(level)
|
|
{
|
|
}
|
|
virtual ~Heading() override = default;
|
|
virtual ByteString render_to_html() const override;
|
|
|
|
private:
|
|
int m_level { 1 };
|
|
};
|
|
|
|
}
|