mirror of
https://github.com/RGBCube/serenity
synced 2025-07-05 21:27:35 +00:00
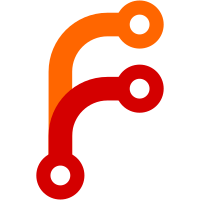
Previously, a libc-like out-of-line error information was used in the loader and its plugins. Now, all functions that may fail to do their job return some sort of Result. The universally-used error type ist the new LoaderError, which can contain information about the general error category (such as file format, I/O, unimplemented features), an error description, and location information, such as file index or sample index. Additionally, the loader plugins try to do as little work as possible in their constructors. Right after being constructed, a user should call initialize() and check the errors returned from there. (This is done transparently by Loader itself.) If a constructor caused an error, the call to initialize should check and return it immediately. This opportunity was used to rework a lot of the internal error propagation in both loader classes, especially FlacLoader. Therefore, a couple of other refactorings may have sneaked in as well. The adoption of LibAudio users is minimal. Piano's adoption is not important, as the code will receive major refactoring in the near future anyways. SoundPlayer's adoption is also less important, as changes to refactor it are in the works as well. aplay's adoption is the best and may serve as an example for other users. It also includes new buffering behavior. Buffer also gets some attention, making it OOM-safe and thereby also propagating its errors to the user.
75 lines
2.4 KiB
C++
75 lines
2.4 KiB
C++
/*
|
|
* Copyright (c) 2018-2020, Andreas Kling <kling@serenityos.org>
|
|
* Copyright (c) 2021, kleines Filmröllchen <malu.bertsch@gmail.com>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <AK/ByteBuffer.h>
|
|
#include <AK/MemoryStream.h>
|
|
#include <AK/OwnPtr.h>
|
|
#include <AK/RefPtr.h>
|
|
#include <AK/Stream.h>
|
|
#include <AK/String.h>
|
|
#include <AK/StringView.h>
|
|
#include <AK/WeakPtr.h>
|
|
#include <LibAudio/Buffer.h>
|
|
#include <LibAudio/Loader.h>
|
|
#include <LibCore/File.h>
|
|
#include <LibCore/FileStream.h>
|
|
|
|
namespace Audio {
|
|
class Buffer;
|
|
|
|
// defines for handling the WAV header data
|
|
#define WAVE_FORMAT_PCM 0x0001 // PCM
|
|
#define WAVE_FORMAT_IEEE_FLOAT 0x0003 // IEEE float
|
|
#define WAVE_FORMAT_ALAW 0x0006 // 8-bit ITU-T G.711 A-law
|
|
#define WAVE_FORMAT_MULAW 0x0007 // 8-bit ITU-T G.711 µ-law
|
|
#define WAVE_FORMAT_EXTENSIBLE 0xFFFE // Determined by SubFormat
|
|
|
|
// Parses a WAV file and produces an Audio::Buffer.
|
|
class WavLoaderPlugin : public LoaderPlugin {
|
|
public:
|
|
explicit WavLoaderPlugin(StringView path);
|
|
explicit WavLoaderPlugin(const ByteBuffer& buffer);
|
|
|
|
virtual MaybeLoaderError initialize() override;
|
|
|
|
// The Buffer returned contains input data resampled at the
|
|
// destination audio device sample rate.
|
|
virtual LoaderSamples get_more_samples(size_t max_bytes_to_read_from_input = 128 * KiB) override;
|
|
|
|
virtual MaybeLoaderError reset() override { return seek(0); }
|
|
|
|
// sample_index 0 is the start of the raw audio sample data
|
|
// within the file/stream.
|
|
virtual MaybeLoaderError seek(const int sample_index) override;
|
|
|
|
virtual int loaded_samples() override { return m_loaded_samples; }
|
|
virtual int total_samples() override { return m_total_samples; }
|
|
virtual u32 sample_rate() override { return m_sample_rate; }
|
|
virtual u16 num_channels() override { return m_num_channels; }
|
|
virtual PcmSampleFormat pcm_format() override { return m_sample_format; }
|
|
virtual RefPtr<Core::File> file() override { return m_file; }
|
|
|
|
private:
|
|
MaybeLoaderError parse_header();
|
|
|
|
RefPtr<Core::File> m_file;
|
|
OwnPtr<AK::InputStream> m_stream;
|
|
AK::InputMemoryStream* m_memory_stream;
|
|
Optional<LoaderError> m_error {};
|
|
|
|
u32 m_sample_rate { 0 };
|
|
u16 m_num_channels { 0 };
|
|
PcmSampleFormat m_sample_format;
|
|
size_t m_byte_offset_of_data_samples { 0 };
|
|
|
|
int m_loaded_samples { 0 };
|
|
int m_total_samples { 0 };
|
|
};
|
|
|
|
}
|