mirror of
https://github.com/RGBCube/serenity
synced 2025-05-25 17:25:08 +00:00
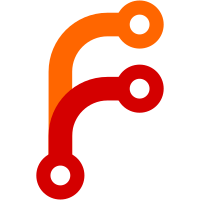
This option is already enabled when building Lagom, so let's enable it for the main build too. We will no longer be surprised by Lagom Clang CI builds failing while everything compiles locally. Furthermore, the stronger `-Wsuggest-override` warning is enabled in this commit, which enforces the use of the `override` keyword in all classes, not just those which already have some methods marked as `override`. This works with both GCC and Clang.
65 lines
1.1 KiB
C++
65 lines
1.1 KiB
C++
/*
|
|
* Copyright (c) 2020, the SerenityOS developers.
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <AK/Span.h>
|
|
#include <AK/Types.h>
|
|
#include <LibCrypto/Checksum/ChecksumFunction.h>
|
|
|
|
namespace Crypto::Checksum {
|
|
|
|
struct Table {
|
|
u32 data[256];
|
|
|
|
constexpr Table()
|
|
: data()
|
|
{
|
|
for (auto i = 0; i < 256; i++) {
|
|
u32 value = i;
|
|
|
|
for (auto j = 0; j < 8; j++) {
|
|
if (value & 1) {
|
|
value = 0xEDB88320 ^ (value >> 1);
|
|
} else {
|
|
value = value >> 1;
|
|
}
|
|
}
|
|
|
|
data[i] = value;
|
|
}
|
|
}
|
|
|
|
constexpr u32 operator[](int index) const
|
|
{
|
|
return data[index];
|
|
}
|
|
};
|
|
|
|
constexpr static auto table = Table();
|
|
|
|
class CRC32 : public ChecksumFunction<u32> {
|
|
public:
|
|
CRC32() { }
|
|
CRC32(ReadonlyBytes data)
|
|
{
|
|
update(data);
|
|
}
|
|
|
|
CRC32(u32 initial_state, ReadonlyBytes data)
|
|
: m_state(initial_state)
|
|
{
|
|
update(data);
|
|
}
|
|
|
|
virtual void update(ReadonlyBytes data) override;
|
|
virtual u32 digest() override;
|
|
|
|
private:
|
|
u32 m_state { ~0u };
|
|
};
|
|
|
|
}
|