mirror of
https://github.com/RGBCube/serenity
synced 2025-05-14 08:54:58 +00:00
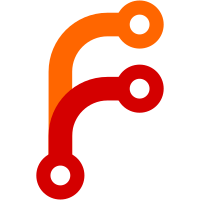
Remove all functions with platform #if's from fenv, and add arch dependent implementations instead. The build system now selects the implementation based on the platform.
108 lines
1.5 KiB
C++
108 lines
1.5 KiB
C++
/*
|
|
* Copyright (c) 2024, Tom Finet <tom.codeninja@gmail.com>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#include <AK/Types.h>
|
|
#include <fenv.h>
|
|
|
|
extern "C" {
|
|
|
|
int fegetenv(fenv_t* env)
|
|
{
|
|
if (!env)
|
|
return 1;
|
|
|
|
(void)env;
|
|
TODO_RISCV64();
|
|
return 0;
|
|
}
|
|
|
|
int fesetenv(fenv_t const* env)
|
|
{
|
|
if (!env)
|
|
return 1;
|
|
|
|
(void)env;
|
|
TODO_RISCV64();
|
|
return 0;
|
|
}
|
|
|
|
int feholdexcept(fenv_t* env)
|
|
{
|
|
fegetenv(env);
|
|
|
|
fenv_t current_env;
|
|
fegetenv(¤t_env);
|
|
|
|
(void)env;
|
|
TODO_RISCV64();
|
|
|
|
fesetenv(¤t_env);
|
|
return 0;
|
|
}
|
|
|
|
int fesetexceptflag(fexcept_t const* except, int exceptions)
|
|
{
|
|
if (!except)
|
|
return 1;
|
|
|
|
fenv_t current_env;
|
|
fegetenv(¤t_env);
|
|
|
|
exceptions &= FE_ALL_EXCEPT;
|
|
|
|
(void)exceptions;
|
|
(void)except;
|
|
TODO_RISCV64();
|
|
|
|
fesetenv(¤t_env);
|
|
return 0;
|
|
}
|
|
|
|
int fegetround()
|
|
{
|
|
TODO_RISCV64();
|
|
}
|
|
|
|
int fesetround(int rounding_mode)
|
|
{
|
|
if (rounding_mode < FE_TONEAREST || rounding_mode > FE_TOWARDZERO)
|
|
return 1;
|
|
|
|
TODO_RISCV64();
|
|
return 0;
|
|
}
|
|
|
|
int feclearexcept(int exceptions)
|
|
{
|
|
exceptions &= FE_ALL_EXCEPT;
|
|
|
|
fenv_t current_env;
|
|
fegetenv(¤t_env);
|
|
|
|
(void)exceptions;
|
|
TODO_RISCV64();
|
|
|
|
fesetenv(¤t_env);
|
|
return 0;
|
|
}
|
|
|
|
int fetestexcept(int exceptions)
|
|
{
|
|
(void)exceptions;
|
|
TODO_RISCV64();
|
|
}
|
|
|
|
int feraiseexcept(int exceptions)
|
|
{
|
|
fenv_t env;
|
|
fegetenv(&env);
|
|
|
|
exceptions &= FE_ALL_EXCEPT;
|
|
|
|
(void)exceptions;
|
|
TODO_RISCV64();
|
|
}
|
|
}
|