mirror of
https://github.com/RGBCube/serenity
synced 2025-07-04 12:07:35 +00:00
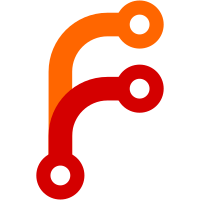
Also added a local test for ensuring this behavior since it is unique to browsers. Since we don't actually use WindowProxy anywhere yet we just test on location for now.
63 lines
2.1 KiB
HTML
63 lines
2.1 KiB
HTML
<html>
|
|
<body>
|
|
<span
|
|
>If passed, the text below should say: "Passed" And the iframe should have loaded</span
|
|
>
|
|
<div id="result">Starting up</div>
|
|
<script>
|
|
class Base {
|
|
constructor(o) {
|
|
return o;
|
|
}
|
|
}
|
|
|
|
class Stamper extends Base {
|
|
#x = 10;
|
|
static hasX(o) {
|
|
return #x in o;
|
|
}
|
|
}
|
|
|
|
let failed = false;
|
|
function status(text, fail = false) {
|
|
console.log("Status", text, fail, failed);
|
|
const output = document.getElementById("result");
|
|
if (fail) {
|
|
output.innerHTML += "Fail: " + text + "<br />";
|
|
output.style.color = "red";
|
|
failed = true;
|
|
} else if (!failed) {
|
|
output.innerHTML = text;
|
|
output.style.color = "blue";
|
|
}
|
|
}
|
|
|
|
function fail(text) {
|
|
return status(text, true);
|
|
}
|
|
|
|
status("Running");
|
|
|
|
const iframe = document.body.appendChild(document.createElement("iframe"));
|
|
iframe.src = "file:///res/html/misc/welcome.html";
|
|
iframe.onload = () => {
|
|
status("Inside onload", false);
|
|
// FIXME: Also test on contentWindow itself once that is an actual WindowProxy.
|
|
const locationObject = iframe.contentWindow.location;
|
|
try {
|
|
new Stamper(locationObject);
|
|
fail("Should have thrown type error!");
|
|
} catch (e) {
|
|
if (!(e instanceof TypeError))
|
|
fail("Should have thrown type error! But threw: " + e);
|
|
}
|
|
if (Stamper.hasX(locationObject)) {
|
|
fail("Stamped #x on Location");
|
|
} else {
|
|
status("Passed");
|
|
}
|
|
};
|
|
status("Set src and onload, waiting for onload");
|
|
</script>
|
|
</body>
|
|
</html>
|