mirror of
https://github.com/RGBCube/serenity
synced 2025-05-16 23:15:07 +00:00
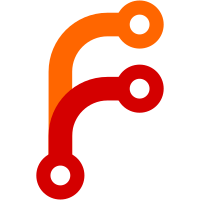
Instead of crashing with a TODO() on half of the test cases generated by Domato, let's just return a zeroed-out SVGAnimatedLength or SVGAnimatedNumber from getters that return them. We'll eventually have to implement these correctly, but crashing is not productive since it blocks us from finding other issues.
75 lines
2.4 KiB
C++
75 lines
2.4 KiB
C++
/*
|
|
* Copyright (c) 2023, MacDue <macdue@dueutil.tech>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#include <LibWeb/Bindings/Intrinsics.h>
|
|
#include <LibWeb/CSS/Parser/Parser.h>
|
|
#include <LibWeb/CSS/StyleValues/IdentifierStyleValue.h>
|
|
#include <LibWeb/Layout/BlockContainer.h>
|
|
#include <LibWeb/SVG/AttributeNames.h>
|
|
#include <LibWeb/SVG/AttributeParser.h>
|
|
#include <LibWeb/SVG/SVGStopElement.h>
|
|
|
|
namespace Web::SVG {
|
|
|
|
JS_DEFINE_ALLOCATOR(SVGStopElement);
|
|
|
|
SVGStopElement::SVGStopElement(DOM::Document& document, DOM::QualifiedName qualified_name)
|
|
: SVGElement(document, qualified_name)
|
|
{
|
|
}
|
|
|
|
void SVGStopElement::attribute_changed(FlyString const& name, Optional<String> const& value)
|
|
{
|
|
SVGElement::attribute_changed(name, value);
|
|
if (name == SVG::AttributeNames::offset) {
|
|
m_offset = AttributeParser::parse_number_percentage(value.value_or(String {}));
|
|
}
|
|
}
|
|
|
|
void SVGStopElement::apply_presentational_hints(CSS::StyleProperties& style) const
|
|
{
|
|
CSS::Parser::ParsingContext parsing_context { document() };
|
|
for_each_attribute([&](auto& name, auto& value) {
|
|
CSS::Parser::ParsingContext parsing_context { document() };
|
|
if (name.equals_ignoring_ascii_case("stop-color"sv)) {
|
|
if (auto stop_color = parse_css_value(parsing_context, value, CSS::PropertyID::StopColor)) {
|
|
style.set_property(CSS::PropertyID::StopColor, stop_color.release_nonnull());
|
|
}
|
|
} else if (name.equals_ignoring_ascii_case("stop-opacity"sv)) {
|
|
if (auto stop_opacity = parse_css_value(parsing_context, value, CSS::PropertyID::StopOpacity)) {
|
|
style.set_property(CSS::PropertyID::StopOpacity, stop_opacity.release_nonnull());
|
|
}
|
|
}
|
|
});
|
|
}
|
|
|
|
Gfx::Color SVGStopElement::stop_color() const
|
|
{
|
|
if (auto css_values = computed_css_values())
|
|
return css_values->stop_color();
|
|
return Color::Black;
|
|
}
|
|
|
|
float SVGStopElement::stop_opacity() const
|
|
{
|
|
if (auto css_values = computed_css_values())
|
|
return css_values->stop_opacity();
|
|
return 1;
|
|
}
|
|
|
|
JS::NonnullGCPtr<SVGAnimatedNumber> SVGStopElement::offset() const
|
|
{
|
|
// FIXME: Implement this properly.
|
|
return SVGAnimatedNumber::create(realm(), 0, 0);
|
|
}
|
|
|
|
void SVGStopElement::initialize(JS::Realm& realm)
|
|
{
|
|
Base::initialize(realm);
|
|
set_prototype(&Bindings::ensure_web_prototype<Bindings::SVGStopElementPrototype>(realm, "SVGStopElement"_fly_string));
|
|
}
|
|
|
|
}
|