mirror of
https://github.com/RGBCube/serenity
synced 2025-05-14 11:34:59 +00:00
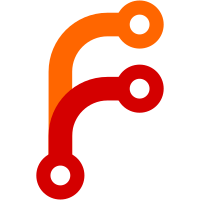
Previously the argument order for Margins was (left, top, right, bottom). To make it more familiar and closer to how CSS does it, the argument order is now (top, right, bottom, left).
106 lines
3.5 KiB
C++
106 lines
3.5 KiB
C++
/*
|
|
* Copyright (c) 2018-2020, Andreas Kling <kling@serenityos.org>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#include <AK/StringBuilder.h>
|
|
#include <LibCore/ConfigFile.h>
|
|
#include <LibGUI/AboutDialog.h>
|
|
#include <LibGUI/BoxLayout.h>
|
|
#include <LibGUI/Button.h>
|
|
#include <LibGUI/ImageWidget.h>
|
|
#include <LibGUI/Label.h>
|
|
#include <LibGUI/Widget.h>
|
|
#include <LibGfx/Font.h>
|
|
#include <LibGfx/FontDatabase.h>
|
|
|
|
namespace GUI {
|
|
|
|
AboutDialog::AboutDialog(const StringView& name, const Gfx::Bitmap* icon, Window* parent_window)
|
|
: Dialog(parent_window)
|
|
, m_name(name)
|
|
, m_icon(icon)
|
|
{
|
|
resize(413, 204);
|
|
set_title(String::formatted("About {}", m_name));
|
|
set_resizable(false);
|
|
|
|
if (parent_window)
|
|
set_icon(parent_window->icon());
|
|
|
|
auto& widget = set_main_widget<Widget>();
|
|
widget.set_fill_with_background_color(true);
|
|
widget.set_layout<VerticalBoxLayout>();
|
|
widget.layout()->set_spacing(0);
|
|
|
|
auto& banner_image = widget.add<GUI::ImageWidget>();
|
|
banner_image.load_from_file("/res/graphics/brand-banner.png");
|
|
|
|
auto& content_container = widget.add<Widget>();
|
|
content_container.set_layout<HorizontalBoxLayout>();
|
|
|
|
auto& left_container = content_container.add<Widget>();
|
|
left_container.set_fixed_width(60);
|
|
left_container.set_layout<VerticalBoxLayout>();
|
|
left_container.layout()->set_margins({ 12, 0, 0, 0 });
|
|
|
|
if (icon) {
|
|
auto& icon_wrapper = left_container.add<Widget>();
|
|
icon_wrapper.set_fixed_size(32, 48);
|
|
icon_wrapper.set_layout<VerticalBoxLayout>();
|
|
|
|
auto& icon_image = icon_wrapper.add<ImageWidget>();
|
|
icon_image.set_bitmap(m_icon);
|
|
}
|
|
|
|
auto& right_container = content_container.add<Widget>();
|
|
right_container.set_layout<VerticalBoxLayout>();
|
|
right_container.layout()->set_margins({ 12, 4, 4, 0 });
|
|
|
|
auto make_label = [&](const StringView& text, bool bold = false) {
|
|
auto& label = right_container.add<Label>(text);
|
|
label.set_text_alignment(Gfx::TextAlignment::CenterLeft);
|
|
label.set_fixed_height(14);
|
|
label.set_content_margins({ 0, 8, 0, 0 });
|
|
if (bold)
|
|
label.set_font(Gfx::FontDatabase::default_font().bold_variant());
|
|
};
|
|
make_label(m_name, true);
|
|
// If we are displaying a dialog for an application, insert 'SerenityOS' below the application name
|
|
if (m_name != "SerenityOS")
|
|
make_label("SerenityOS");
|
|
make_label(version_string());
|
|
make_label("Copyright \xC2\xA9 the SerenityOS developers, 2018-2021");
|
|
|
|
right_container.layout()->add_spacer();
|
|
|
|
auto& button_container = right_container.add<Widget>();
|
|
button_container.set_fixed_height(22);
|
|
button_container.set_layout<HorizontalBoxLayout>();
|
|
button_container.layout()->add_spacer();
|
|
auto& ok_button = button_container.add<Button>("OK");
|
|
ok_button.set_fixed_width(80);
|
|
ok_button.on_click = [this](auto) {
|
|
done(Dialog::ExecOK);
|
|
};
|
|
}
|
|
|
|
AboutDialog::~AboutDialog()
|
|
{
|
|
}
|
|
|
|
String AboutDialog::version_string() const
|
|
{
|
|
auto version_config = Core::ConfigFile::open("/res/version.ini");
|
|
auto major_version = version_config->read_entry("Version", "Major", "0");
|
|
auto minor_version = version_config->read_entry("Version", "Minor", "0");
|
|
|
|
StringBuilder builder;
|
|
builder.appendff("Version {}.{}", major_version, minor_version);
|
|
if (auto git_version = version_config->read_entry("Version", "Git", ""); git_version != "")
|
|
builder.appendff(".g{}", git_version);
|
|
return builder.to_string();
|
|
}
|
|
|
|
}
|