mirror of
https://github.com/RGBCube/serenity
synced 2025-07-09 12:17:35 +00:00
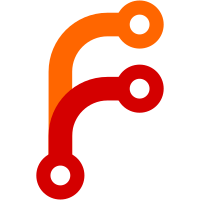
Globally shared MemoryManager state is now kept in a GlobalData struct and wrapped in SpinlockProtected. A small set of members are left outside the GlobalData struct as they are only set during boot initialization, and then remain constant. This allows us to access those members without taking any locks.
67 lines
1.8 KiB
C++
67 lines
1.8 KiB
C++
/*
|
|
* Copyright (c) 2021, the SerenityOS developers.
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#include <AK/OwnPtr.h>
|
|
#include <AK/Types.h>
|
|
#include <Kernel/Library/LockRefPtr.h>
|
|
#include <Kernel/Storage/Ramdisk/Controller.h>
|
|
|
|
namespace Kernel {
|
|
|
|
NonnullLockRefPtr<RamdiskController> RamdiskController::initialize()
|
|
{
|
|
return adopt_lock_ref(*new RamdiskController());
|
|
}
|
|
|
|
bool RamdiskController::reset()
|
|
{
|
|
TODO();
|
|
}
|
|
|
|
bool RamdiskController::shutdown()
|
|
{
|
|
TODO();
|
|
}
|
|
|
|
size_t RamdiskController::devices_count() const
|
|
{
|
|
return m_devices.size();
|
|
}
|
|
|
|
void RamdiskController::complete_current_request(AsyncDeviceRequest::RequestResult)
|
|
{
|
|
VERIFY_NOT_REACHED();
|
|
}
|
|
|
|
RamdiskController::RamdiskController()
|
|
: StorageController()
|
|
{
|
|
// Populate ramdisk controllers from Multiboot boot modules, if any.
|
|
size_t count = 0;
|
|
MM.for_each_used_memory_range([&](auto& used_memory_range) {
|
|
if (used_memory_range.type == Memory::UsedMemoryRangeType::BootModule) {
|
|
size_t length = Memory::page_round_up(used_memory_range.end.get()).release_value_but_fixme_should_propagate_errors() - used_memory_range.start.get();
|
|
auto region_or_error = MM.allocate_kernel_region(used_memory_range.start, length, "Ramdisk"sv, Memory::Region::Access::ReadWrite);
|
|
if (region_or_error.is_error()) {
|
|
dmesgln("RamdiskController: Failed to allocate kernel region of size {}", length);
|
|
} else {
|
|
m_devices.append(RamdiskDevice::create(*this, region_or_error.release_value(), 6, count));
|
|
}
|
|
count++;
|
|
}
|
|
});
|
|
}
|
|
|
|
RamdiskController::~RamdiskController() = default;
|
|
|
|
LockRefPtr<StorageDevice> RamdiskController::device(u32 index) const
|
|
{
|
|
if (index >= m_devices.size())
|
|
return nullptr;
|
|
return m_devices[index];
|
|
}
|
|
|
|
}
|