mirror of
https://github.com/RGBCube/serenity
synced 2025-07-01 22:32:07 +00:00
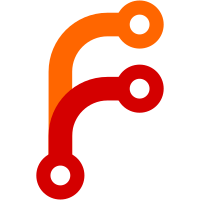
This is a partial revert of commit7af5eef
. After97d15e9
, the 'proc' promise is not needed for operations using getsid(). This also fixes launching several applications in which7af5eef
added the 'proc' promise only in the second call to pledge().
86 lines
2.9 KiB
C++
86 lines
2.9 KiB
C++
/*
|
|
* Copyright (c) 2018-2021, Andreas Kling <kling@serenityos.org>
|
|
* Copyright (c) 2021, Mustafa Quraish <mustafa@serenityos.org>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#include "MainWidget.h"
|
|
#include <LibConfig/Client.h>
|
|
#include <LibCore/ArgsParser.h>
|
|
#include <LibCore/System.h>
|
|
#include <LibFileSystemAccessClient/Client.h>
|
|
#include <LibGUI/Action.h>
|
|
#include <LibGUI/Application.h>
|
|
#include <LibGUI/Icon.h>
|
|
#include <LibGUI/MessageBox.h>
|
|
#include <LibGUI/Statusbar.h>
|
|
#include <LibGUI/Window.h>
|
|
#include <LibGfx/Painter.h>
|
|
#include <LibMain/Main.h>
|
|
|
|
ErrorOr<int> serenity_main(Main::Arguments arguments)
|
|
{
|
|
TRY(Core::System::pledge("stdio thread recvfd sendfd rpath unix wpath cpath"));
|
|
|
|
auto app = TRY(GUI::Application::try_create(arguments));
|
|
Config::pledge_domain("PixelPaint");
|
|
|
|
char const* image_file = nullptr;
|
|
Core::ArgsParser args_parser;
|
|
args_parser.add_positional_argument(image_file, "Image file to open", "path", Core::ArgsParser::Required::No);
|
|
args_parser.parse(arguments);
|
|
|
|
TRY(Core::System::unveil("/proc/all", "r"));
|
|
TRY(Core::System::unveil("/res", "r"));
|
|
TRY(Core::System::unveil("/tmp/portal/clipboard", "rw"));
|
|
TRY(Core::System::unveil("/tmp/session/%sid/portal/filesystemaccess", "rw"));
|
|
TRY(Core::System::unveil("/tmp/session/%sid/portal/image", "rw"));
|
|
TRY(Core::System::unveil("/etc/FileIconProvider.ini", "r"));
|
|
TRY(Core::System::unveil(nullptr, nullptr));
|
|
|
|
auto app_icon = GUI::Icon::default_icon("app-pixel-paint"sv);
|
|
|
|
PixelPaint::g_icon_bag = TRY(PixelPaint::IconBag::try_create());
|
|
|
|
auto window = GUI::Window::construct();
|
|
window->set_title("Pixel Paint");
|
|
window->resize(800, 510);
|
|
window->set_icon(app_icon.bitmap_for_size(16));
|
|
|
|
auto main_widget = TRY(window->try_set_main_widget<PixelPaint::MainWidget>());
|
|
|
|
main_widget->initialize_menubar(*window);
|
|
|
|
window->on_close_request = [&]() -> GUI::Window::CloseRequestDecision {
|
|
if (main_widget->request_close())
|
|
return GUI::Window::CloseRequestDecision::Close;
|
|
return GUI::Window::CloseRequestDecision::StayOpen;
|
|
};
|
|
|
|
auto& statusbar = *main_widget->find_descendant_of_type_named<GUI::Statusbar>("statusbar");
|
|
|
|
app->on_action_enter = [&statusbar](GUI::Action& action) {
|
|
auto text = action.status_tip();
|
|
if (text.is_empty())
|
|
text = Gfx::parse_ampersand_string(action.text());
|
|
statusbar.set_override_text(move(text));
|
|
};
|
|
|
|
app->on_action_leave = [&statusbar](GUI::Action&) {
|
|
statusbar.set_override_text({});
|
|
};
|
|
|
|
window->show();
|
|
|
|
if (image_file) {
|
|
auto response = FileSystemAccessClient::Client::the().try_request_file_read_only_approved(window, image_file);
|
|
if (response.is_error())
|
|
return 1;
|
|
main_widget->open_image(response.value());
|
|
} else {
|
|
main_widget->create_default_image();
|
|
}
|
|
|
|
return app->exec();
|
|
}
|