mirror of
https://github.com/RGBCube/serenity
synced 2025-05-22 08:45:09 +00:00
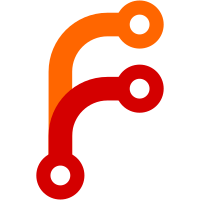
There's basically no real difference in software between a SATA harddisk and IDE harddisk. The difference in the implementation is for the host bus adapter protocol and registers layout. Therefore, there's no point in putting a distinction in software to these devices. This change also greatly simplifies and removes stale APIs and removes unnecessary parameters in constructor calls, which tighten things further everywhere.
46 lines
1.3 KiB
C++
46 lines
1.3 KiB
C++
/*
|
|
* Copyright (c) 2021, Liav A. <liavalb@hotmail.co.il>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <Kernel/Interrupts/IRQHandler.h>
|
|
#include <Kernel/Locking/Mutex.h>
|
|
#include <Kernel/Storage/ATAController.h>
|
|
#include <Kernel/Storage/StorageDevice.h>
|
|
|
|
namespace Kernel {
|
|
|
|
class ATADevice : public StorageDevice {
|
|
public:
|
|
// Note: For IDE drives, port means Primary or Secondary (0 or 1),
|
|
// and subport means Master or Slave (0 or 1).
|
|
// For SATA drives (AHCI driven HBAs), a port can be a number from 0 to 31,
|
|
// and subport can be a number from 0 to 14 (only 15 devices are allowed to
|
|
// be connected to one SATA port multiplier).
|
|
struct Address {
|
|
// FIXME: u32 for this value is wasteful, because even AHCI only support 32 ports
|
|
u32 port;
|
|
u8 subport;
|
|
};
|
|
|
|
public:
|
|
virtual ~ATADevice() override;
|
|
|
|
// ^BlockDevice
|
|
virtual void start_request(AsyncBlockDeviceRequest&) override;
|
|
|
|
u16 ata_capabilites() const { return m_capabilities; }
|
|
const Address& ata_address() const { return m_ata_address; }
|
|
|
|
protected:
|
|
ATADevice(const ATAController&, Address, unsigned, u16, u16, u64, NonnullOwnPtr<KString>);
|
|
|
|
WeakPtr<ATAController> m_controller;
|
|
const Address m_ata_address;
|
|
const u16 m_capabilities;
|
|
};
|
|
|
|
}
|