mirror of
https://github.com/RGBCube/serenity
synced 2025-07-12 14:07:35 +00:00
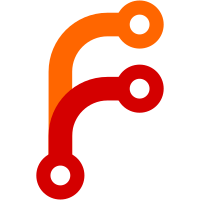
This adds the option to pass a subpixel offset when fetching a glyph from a font, this offset is currently snapped to thirds of a pixel (i.e. 0, 0.33, 0.66). This is then used when rasterizing the glyph, which is then cached like usual. Note that when using subpixel offsets you're trading a bit of space for accuracy. With the current third of a pixel offsets you can end up with up to 9 bitmaps per glyph.
33 lines
666 B
C++
33 lines
666 B
C++
/*
|
|
* Copyright (c) 2020, Srimanta Barua <srimanta.barua1@gmail.com>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <AK/Vector.h>
|
|
#include <LibGfx/Bitmap.h>
|
|
#include <LibGfx/Path.h>
|
|
|
|
namespace Gfx {
|
|
|
|
class PathRasterizer {
|
|
public:
|
|
PathRasterizer(Gfx::IntSize);
|
|
void draw_path(Gfx::Path&);
|
|
RefPtr<Gfx::Bitmap> accumulate();
|
|
|
|
void translate(Gfx::FloatPoint delta) { m_translation.translate_by(delta); }
|
|
Gfx::FloatPoint translation() const { return m_translation; }
|
|
|
|
private:
|
|
void draw_line(Gfx::FloatPoint, Gfx::FloatPoint);
|
|
|
|
Gfx::IntSize m_size;
|
|
Gfx::FloatPoint m_translation;
|
|
|
|
Vector<float> m_data;
|
|
};
|
|
|
|
}
|