mirror of
https://github.com/RGBCube/serenity
synced 2025-07-05 10:47:36 +00:00
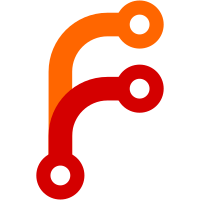
The absolute rect of a paintable is somewhat expensive to compute. This is because all coordinates are relative to the nearest containing block, so we have to traverse the containing block chain and apply each offset to get the final rect. Paintables will never move between containing blocks, nor will their absolute rect change. If anything changes, we'll simpl make a new paintable and replace the old one. Take advantage of this by caching the containing block and absolute rect after first access.
46 lines
1.3 KiB
C++
46 lines
1.3 KiB
C++
/*
|
|
* Copyright (c) 2022, Andreas Kling <kling@serenityos.org>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#include <LibWeb/DOM/Document.h>
|
|
#include <LibWeb/Layout/BlockContainer.h>
|
|
#include <LibWeb/Painting/Paintable.h>
|
|
|
|
namespace Web::Painting {
|
|
|
|
void Paintable::handle_mousedown(Badge<EventHandler>, Gfx::IntPoint const&, unsigned, unsigned)
|
|
{
|
|
}
|
|
|
|
void Paintable::handle_mouseup(Badge<EventHandler>, Gfx::IntPoint const&, unsigned, unsigned)
|
|
{
|
|
}
|
|
|
|
void Paintable::handle_mousemove(Badge<EventHandler>, Gfx::IntPoint const&, unsigned, unsigned)
|
|
{
|
|
}
|
|
|
|
bool Paintable::handle_mousewheel(Badge<EventHandler>, Gfx::IntPoint const&, unsigned, unsigned, int wheel_delta_x, int wheel_delta_y)
|
|
{
|
|
if (auto* containing_block = this->containing_block()) {
|
|
if (!containing_block->is_scrollable())
|
|
return false;
|
|
auto new_offset = containing_block->scroll_offset();
|
|
new_offset.translate_by(wheel_delta_x, wheel_delta_y);
|
|
// FIXME: This const_cast is gross.
|
|
// FIXME: Scroll offset shouldn't live in the layout tree.
|
|
const_cast<Layout::BlockContainer*>(containing_block)->set_scroll_offset(new_offset);
|
|
return true;
|
|
}
|
|
|
|
return false;
|
|
}
|
|
|
|
HitTestResult Paintable::hit_test(Gfx::IntPoint const&, HitTestType) const
|
|
{
|
|
VERIFY_NOT_REACHED();
|
|
}
|
|
|
|
}
|