mirror of
https://github.com/RGBCube/serenity
synced 2025-07-12 06:17:34 +00:00
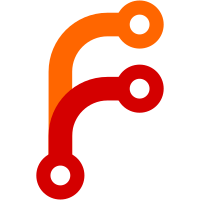
This allows the host of LibJS (notably LibWeb in this case) to override certain functions such as HostEnqueuePromiseJob, so it can do it's own thing in certain situations. Notably, LibWeb will override HostEnqueuePromiseJob to put promise jobs on the microtask queue. This also makes promise jobs use AK::Function instead of JS::NativeFunction. This removes the need to go through a JavaScript function and it more closely matches the spec's idea of "abstract closures"
54 lines
1.5 KiB
C++
54 lines
1.5 KiB
C++
/*
|
|
* Copyright (c) 2021, Idan Horowitz <idan.horowitz@serenityos.org>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <AK/SinglyLinkedList.h>
|
|
#include <LibJS/Runtime/FunctionObject.h>
|
|
#include <LibJS/Runtime/GlobalObject.h>
|
|
#include <LibJS/Runtime/JobCallback.h>
|
|
#include <LibJS/Runtime/Object.h>
|
|
#include <LibJS/Runtime/Value.h>
|
|
#include <LibJS/Runtime/WeakContainer.h>
|
|
|
|
namespace JS {
|
|
|
|
class FinalizationRegistry final
|
|
: public Object
|
|
, public WeakContainer {
|
|
JS_OBJECT(FinalizationRegistry, Object);
|
|
|
|
public:
|
|
explicit FinalizationRegistry(Realm&, JS::JobCallback, Object& prototype);
|
|
virtual ~FinalizationRegistry() override;
|
|
|
|
void add_finalization_record(Cell& target, Value held_value, Object* unregister_token);
|
|
bool remove_by_token(Object& unregister_token);
|
|
ThrowCompletionOr<void> cleanup(Optional<JobCallback> = {});
|
|
|
|
virtual void remove_dead_cells(Badge<Heap>) override;
|
|
|
|
Realm& realm() { return *m_realm.cell(); }
|
|
Realm const& realm() const { return *m_realm.cell(); }
|
|
|
|
JobCallback& cleanup_callback() { return m_cleanup_callback; }
|
|
JobCallback const& cleanup_callback() const { return m_cleanup_callback; }
|
|
|
|
private:
|
|
virtual void visit_edges(Visitor& visitor) override;
|
|
|
|
Handle<Realm> m_realm;
|
|
JS::JobCallback m_cleanup_callback;
|
|
|
|
struct FinalizationRecord {
|
|
Cell* target { nullptr };
|
|
Value held_value;
|
|
Object* unregister_token { nullptr };
|
|
};
|
|
SinglyLinkedList<FinalizationRecord> m_records;
|
|
};
|
|
|
|
}
|