mirror of
https://github.com/RGBCube/serenity
synced 2025-05-18 01:05:07 +00:00
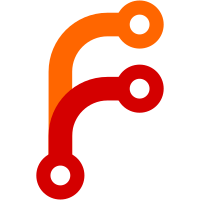
With this patch, it's now possible to pass a Gfx::ShareableBitmap in an IPC message. As long as the message itself is synchronous, the bitmap will be adopted by the receiving end, and disowned by the sender nicely without any accounting effort like we've had to do in the past. Use this in NotificationServer to allow sending arbitrary bitmaps as icons instead of paths-to-icons.
33 lines
671 B
C++
33 lines
671 B
C++
#pragma once
|
|
|
|
#include <LibCore/Object.h>
|
|
#include <LibGfx/Bitmap.h>
|
|
|
|
namespace GUI {
|
|
|
|
class Notification : public Core::Object {
|
|
C_OBJECT(Notification);
|
|
|
|
public:
|
|
virtual ~Notification() override;
|
|
|
|
const String& text() const { return m_text; }
|
|
void set_text(const String& text) { m_text = text; }
|
|
|
|
const String& title() const { return m_title; }
|
|
void set_title(const String& title) { m_title = title; }
|
|
|
|
const Gfx::Bitmap* icon() const { return m_icon; }
|
|
void set_icon(const Gfx::Bitmap* icon) { m_icon = icon; }
|
|
|
|
void show();
|
|
|
|
private:
|
|
Notification();
|
|
|
|
String m_title;
|
|
String m_text;
|
|
RefPtr<Gfx::Bitmap> m_icon;
|
|
};
|
|
|
|
}
|