mirror of
https://github.com/RGBCube/serenity
synced 2025-07-04 02:27:35 +00:00
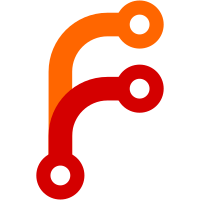
Previously SpinBox did not update on return or changes to the editor. The widget had to lose focus or be manually incremented. This lets the editor update on return and now always displays the most recent clamped value. set_value_from_current_text() will also be useful to programmatically set SpinBox within layouts whose default buttons consume return key presses.
48 lines
1.3 KiB
C++
48 lines
1.3 KiB
C++
/*
|
|
* Copyright (c) 2018-2020, Andreas Kling <kling@serenityos.org>
|
|
* Copyright (c) 2022, the SerenityOS developers.
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <LibGUI/Widget.h>
|
|
|
|
namespace GUI {
|
|
|
|
class SpinBox : public Widget {
|
|
C_OBJECT(SpinBox)
|
|
public:
|
|
virtual ~SpinBox() override = default;
|
|
|
|
int value() const { return m_value; }
|
|
void set_value(int, AllowCallback = AllowCallback::Yes);
|
|
void set_value_from_current_text();
|
|
|
|
int min() const { return m_min; }
|
|
int max() const { return m_max; }
|
|
void set_min(int min, AllowCallback allow_callback = AllowCallback::Yes) { set_range(min, max(), allow_callback); }
|
|
void set_max(int max, AllowCallback allow_callback = AllowCallback::Yes) { set_range(min(), max, allow_callback); }
|
|
void set_range(int min, int max, AllowCallback = AllowCallback::Yes);
|
|
|
|
Function<void(int value)> on_change;
|
|
Function<void()> on_return_pressed;
|
|
|
|
protected:
|
|
SpinBox();
|
|
|
|
virtual void mousewheel_event(MouseEvent&) override;
|
|
virtual void resize_event(ResizeEvent&) override;
|
|
|
|
private:
|
|
RefPtr<TextEditor> m_editor;
|
|
RefPtr<Button> m_increment_button;
|
|
RefPtr<Button> m_decrement_button;
|
|
|
|
int m_min { 0 };
|
|
int m_max { 100 };
|
|
int m_value { 0 };
|
|
};
|
|
|
|
}
|