mirror of
https://github.com/RGBCube/serenity
synced 2025-05-19 00:15:08 +00:00
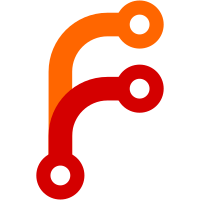
This patch ensures that the shutdown procedure can complete due to the fact we don't kill kernel processes anymore, and only stop the scheduler from running after the filesystems unmount procedure. We also need kernel processes during the shutdown procedure, because we rely on the WorkQueue threads to run WorkQueue items to complete async IO requests initiated by filesystem sync & unmounting, etc. This is also simplifying the code around the killing processes, because we don't need to worry about edge cases such as the FinalizerTask anymore.
38 lines
934 B
C++
38 lines
934 B
C++
/*
|
|
* Copyright (c) 2023, kleines Filmröllchen <filmroellchen@serenityos.org>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <AK/Forward.h>
|
|
#include <Kernel/Forward.h>
|
|
|
|
namespace Kernel {
|
|
|
|
enum class PowerStateCommand : uintptr_t {
|
|
Shutdown,
|
|
Reboot,
|
|
};
|
|
// We will pass the power state command to the task in place of a void* as to avoid the complications of raw allocations.
|
|
static_assert(sizeof(PowerStateCommand) == sizeof(void*));
|
|
|
|
extern bool g_in_system_shutdown;
|
|
|
|
class PowerStateSwitchTask {
|
|
public:
|
|
static void shutdown() { spawn(PowerStateCommand::Shutdown); }
|
|
static void reboot() { spawn(PowerStateCommand::Reboot); }
|
|
|
|
private:
|
|
static void spawn(PowerStateCommand);
|
|
|
|
static void power_state_switch_task(void* raw_entry_data);
|
|
static ErrorOr<void> perform_reboot();
|
|
static ErrorOr<void> perform_shutdown();
|
|
|
|
static ErrorOr<void> kill_all_user_processes();
|
|
};
|
|
|
|
}
|