mirror of
https://github.com/RGBCube/serenity
synced 2025-05-16 14:54:59 +00:00
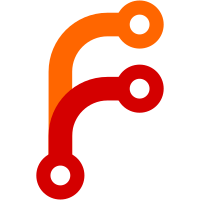
This commit moves the length calculations out to be directly on the StringView users. This is an important step towards the goal of removing StringView(char const*), as it moves the responsibility of calculating the size of the string to the user of the StringView (which will prevent naive uses causing OOB access).
81 lines
1.6 KiB
C++
81 lines
1.6 KiB
C++
/*
|
|
* Copyright (c) 2020, Andreas Kling <kling@serenityos.org>
|
|
* Copyright (c) 2021, the SerenityOS developers.
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <AK/StringView.h>
|
|
|
|
namespace Gfx {
|
|
|
|
struct FontStyleMapping {
|
|
// NOTE: __builtin_strlen required to make this work at compile time.
|
|
constexpr FontStyleMapping(int s, char const* n)
|
|
: style(s)
|
|
, name(StringView { n, __builtin_strlen(n) })
|
|
{
|
|
}
|
|
int style { 0 };
|
|
StringView name;
|
|
};
|
|
|
|
static constexpr FontStyleMapping font_weight_names[] = {
|
|
{ 100, "Thin" },
|
|
{ 200, "Extra Light" },
|
|
{ 300, "Light" },
|
|
{ 400, "Regular" },
|
|
{ 500, "Medium" },
|
|
{ 600, "Semi Bold" },
|
|
{ 700, "Bold" },
|
|
{ 800, "Extra Bold" },
|
|
{ 900, "Black" },
|
|
{ 950, "Extra Black" },
|
|
};
|
|
|
|
static constexpr FontStyleMapping font_slope_names[] = {
|
|
{ 0, "Regular" },
|
|
{ 1, "Italic" },
|
|
{ 2, "Oblique" },
|
|
{ 3, "Reclined" }
|
|
};
|
|
|
|
static constexpr StringView weight_to_name(int weight)
|
|
{
|
|
for (auto& it : font_weight_names) {
|
|
if (it.style == weight)
|
|
return it.name;
|
|
}
|
|
return {};
|
|
}
|
|
|
|
static constexpr int name_to_weight(StringView name)
|
|
{
|
|
for (auto& it : font_weight_names) {
|
|
if (it.name == name)
|
|
return it.style;
|
|
}
|
|
return {};
|
|
}
|
|
|
|
static constexpr StringView slope_to_name(int slope)
|
|
{
|
|
for (auto& it : font_slope_names) {
|
|
if (it.style == slope)
|
|
return it.name;
|
|
}
|
|
return {};
|
|
}
|
|
|
|
static constexpr int name_to_slope(StringView name)
|
|
{
|
|
for (auto& it : font_slope_names) {
|
|
if (it.name == name)
|
|
return it.style;
|
|
}
|
|
return {};
|
|
}
|
|
|
|
}
|