mirror of
https://github.com/RGBCube/serenity
synced 2025-05-18 19:05:06 +00:00
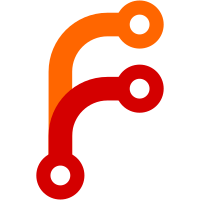
Subclasses of CNetworkJob handle this by overriding shutdown(). This patch implements it for CHttpJob by simply tearing down the underlying socket. We also automatically call shutdown() after the job finishes, regardless of success or failure. :^)
83 lines
1.6 KiB
C++
83 lines
1.6 KiB
C++
#pragma once
|
|
|
|
#include <AK/StdLibExtras.h>
|
|
|
|
// This is a stopgap pointer. It's not meant to stick around forever.
|
|
|
|
template<typename T>
|
|
class ObjectPtr {
|
|
public:
|
|
ObjectPtr() {}
|
|
ObjectPtr(T* ptr)
|
|
: m_ptr(ptr)
|
|
{
|
|
}
|
|
ObjectPtr(T& ptr)
|
|
: m_ptr(&ptr)
|
|
{
|
|
}
|
|
~ObjectPtr()
|
|
{
|
|
if (m_ptr && !m_ptr->parent())
|
|
delete m_ptr;
|
|
}
|
|
|
|
template<typename U>
|
|
ObjectPtr(U* ptr)
|
|
: m_ptr(static_cast<T*>(ptr))
|
|
{
|
|
}
|
|
|
|
ObjectPtr(const ObjectPtr& other)
|
|
: m_ptr(other.m_ptr)
|
|
{
|
|
}
|
|
|
|
template<typename U>
|
|
ObjectPtr(const ObjectPtr<U>& other)
|
|
: m_ptr(static_cast<T*>(const_cast<ObjectPtr<U>&>(other).ptr()))
|
|
{
|
|
}
|
|
|
|
ObjectPtr(ObjectPtr&& other)
|
|
{
|
|
m_ptr = other.leak_ptr();
|
|
}
|
|
|
|
template<typename U>
|
|
ObjectPtr(const ObjectPtr<U>&& other)
|
|
{
|
|
m_ptr = static_cast<T*>(const_cast<ObjectPtr<U>&>(other).leak_ptr());
|
|
}
|
|
|
|
ObjectPtr& operator=(const ObjectPtr& other)
|
|
{
|
|
m_ptr = other.m_ptr;
|
|
return *this;
|
|
}
|
|
|
|
ObjectPtr& operator=(ObjectPtr&& other)
|
|
{
|
|
if (this != &other) {
|
|
m_ptr = exchange(other.m_ptr, nullptr);
|
|
}
|
|
return *this;
|
|
}
|
|
|
|
T* operator->() { return m_ptr; }
|
|
const T* operator->() const { return m_ptr; }
|
|
|
|
operator T*() { return m_ptr; }
|
|
operator const T*() const { return m_ptr; }
|
|
|
|
T& operator*() { return *m_ptr; }
|
|
const T& operator*() const { return *m_ptr; }
|
|
|
|
T* ptr() const { return m_ptr; }
|
|
T* leak_ptr() { return exchange(m_ptr, nullptr); }
|
|
|
|
operator bool() const { return !!m_ptr; }
|
|
|
|
private:
|
|
T* m_ptr { nullptr };
|
|
};
|