mirror of
https://github.com/RGBCube/serenity
synced 2025-05-15 03:04:59 +00:00
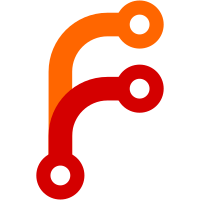
HTMLObjectElement, when implemented according to the spec, does not know the resource type specified by the 'data' attribute until after it has actually loaded (i.e. it may be an image, XML document, etc.). Currently we always use ImageLoader within HTMLObjectElement to load the object, but will need to use ResourceLoader instead to generically load data. However, ImageLoader / ImageResource have image-specific functionality that HTMLObjectElement still needs if the resource turns out to be an image. This patch will allow (only) HTMLObjectElement to convert the generic Resource to an ImageResource as needed.
72 lines
1.7 KiB
C++
72 lines
1.7 KiB
C++
/*
|
|
* Copyright (c) 2020, Andreas Kling <kling@serenityos.org>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <LibWeb/Loader/Resource.h>
|
|
|
|
namespace Web {
|
|
|
|
class ImageResource final : public Resource {
|
|
friend class Resource;
|
|
|
|
public:
|
|
static NonnullRefPtr<ImageResource> convert_from_resource(Resource&);
|
|
|
|
virtual ~ImageResource() override;
|
|
|
|
struct Frame {
|
|
RefPtr<Gfx::Bitmap> bitmap;
|
|
size_t duration { 0 };
|
|
};
|
|
|
|
const Gfx::Bitmap* bitmap(size_t frame_index = 0) const;
|
|
int frame_duration(size_t frame_index) const;
|
|
size_t frame_count() const
|
|
{
|
|
decode_if_needed();
|
|
return m_decoded_frames.size();
|
|
}
|
|
bool is_animated() const
|
|
{
|
|
decode_if_needed();
|
|
return m_animated;
|
|
}
|
|
size_t loop_count() const
|
|
{
|
|
decode_if_needed();
|
|
return m_loop_count;
|
|
}
|
|
|
|
void update_volatility();
|
|
|
|
private:
|
|
explicit ImageResource(const LoadRequest&);
|
|
explicit ImageResource(Resource&);
|
|
|
|
void decode_if_needed() const;
|
|
|
|
mutable bool m_animated { false };
|
|
mutable int m_loop_count { 0 };
|
|
mutable Vector<Frame> m_decoded_frames;
|
|
mutable bool m_has_attempted_decode { false };
|
|
};
|
|
|
|
class ImageResourceClient : public ResourceClient {
|
|
public:
|
|
virtual ~ImageResourceClient();
|
|
|
|
virtual bool is_visible_in_viewport() const { return false; }
|
|
|
|
protected:
|
|
ImageResource* resource() { return static_cast<ImageResource*>(ResourceClient::resource()); }
|
|
const ImageResource* resource() const { return static_cast<const ImageResource*>(ResourceClient::resource()); }
|
|
|
|
private:
|
|
virtual Resource::Type client_type() const override { return Resource::Type::Image; }
|
|
};
|
|
|
|
}
|