mirror of
https://github.com/RGBCube/serenity
synced 2025-05-22 16:55:09 +00:00
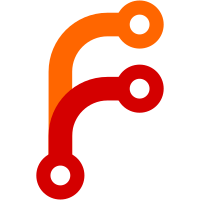
Texture coordinate generation is the concept of automatically generating vertex texture coordinates instead of using the provided coordinates (i.e. `glTexCoord`). This commit implements support for: * The `GL_TEXTURE_GEN_Q/R/S/T` capabilities * The `GL_OBJECT_LINEAR`, `GL_EYE_LINEAR`, `GL_SPHERE_MAP`, `GL_REFLECTION_MAP` and `GL_NORMAL_MAP` modes * Object and eye plane coefficients (write-only at the moment) This changeset allows Tux Racer to render its terrain :^)
88 lines
1.1 KiB
C++
88 lines
1.1 KiB
C++
/*
|
|
* Copyright (c) 2021, Stephan Unverwerth <s.unverwerth@serenityos.org>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
namespace SoftGPU {
|
|
|
|
enum class AlphaTestFunction {
|
|
Never,
|
|
Always,
|
|
Less,
|
|
LessOrEqual,
|
|
Equal,
|
|
NotEqual,
|
|
GreaterOrEqual,
|
|
Greater,
|
|
};
|
|
|
|
enum class BlendFactor {
|
|
Zero,
|
|
One,
|
|
SrcAlpha,
|
|
OneMinusSrcAlpha,
|
|
SrcColor,
|
|
OneMinusSrcColor,
|
|
DstAlpha,
|
|
OneMinusDstAlpha,
|
|
DstColor,
|
|
OneMinusDstColor,
|
|
SrcAlphaSaturate,
|
|
};
|
|
|
|
enum class DepthTestFunction {
|
|
Never,
|
|
Always,
|
|
Less,
|
|
LessOrEqual,
|
|
Equal,
|
|
NotEqual,
|
|
GreaterOrEqual,
|
|
Greater,
|
|
};
|
|
|
|
enum FogMode {
|
|
Linear,
|
|
Exp,
|
|
Exp2
|
|
};
|
|
|
|
enum class PolygonMode {
|
|
Point,
|
|
Line,
|
|
Fill,
|
|
};
|
|
|
|
enum class WindingOrder {
|
|
Clockwise,
|
|
CounterClockwise,
|
|
};
|
|
|
|
enum class PrimitiveType {
|
|
Triangles,
|
|
TriangleStrip,
|
|
TriangleFan,
|
|
Quads,
|
|
};
|
|
|
|
enum TexCoordGenerationCoordinate {
|
|
None = 0x0,
|
|
S = 0x1,
|
|
T = 0x2,
|
|
R = 0x4,
|
|
Q = 0x8,
|
|
All = 0xF,
|
|
};
|
|
|
|
enum class TexCoordGenerationMode {
|
|
ObjectLinear,
|
|
EyeLinear,
|
|
SphereMap,
|
|
ReflectionMap,
|
|
NormalMap,
|
|
};
|
|
|
|
}
|