mirror of
https://github.com/RGBCube/serenity
synced 2025-05-18 11:25:07 +00:00
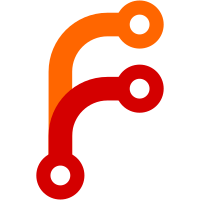
If there's no PCI bus, then it's safe to assume that we run on a x86 machine that has an ISA IDE controller in the system. In such case, we just instantiate a ISAIDEController object that assumes fixed locations of IDE IO ports.
41 lines
1.1 KiB
C++
41 lines
1.1 KiB
C++
/*
|
|
* Copyright (c) 2020-2022, Liav A. <liavalb@hotmail.co.il>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <AK/OwnPtr.h>
|
|
#include <AK/RefPtr.h>
|
|
#include <AK/Types.h>
|
|
#include <Kernel/Storage/ATA/IDEChannel.h>
|
|
#include <Kernel/Storage/ATA/IDEController.h>
|
|
#include <Kernel/Storage/StorageDevice.h>
|
|
|
|
namespace Kernel {
|
|
|
|
class AsyncBlockDeviceRequest;
|
|
|
|
class PCIIDEController final : public IDEController
|
|
, public PCI::Device {
|
|
public:
|
|
static NonnullRefPtr<PCIIDEController> initialize(PCI::DeviceIdentifier const&, bool force_pio);
|
|
|
|
bool is_bus_master_capable() const;
|
|
bool is_pci_native_mode_enabled() const;
|
|
|
|
private:
|
|
bool is_pci_native_mode_enabled_on_primary_channel() const;
|
|
bool is_pci_native_mode_enabled_on_secondary_channel() const;
|
|
PCIIDEController(PCI::DeviceIdentifier const&, bool force_pio);
|
|
|
|
RefPtr<StorageDevice> device_by_channel_and_position(u32 index) const;
|
|
void initialize(bool force_pio);
|
|
void detect_disks();
|
|
|
|
// FIXME: Find a better way to get the ProgrammingInterface
|
|
PCI::ProgrammingInterface m_prog_if;
|
|
PCI::InterruptLine m_interrupt_line;
|
|
};
|
|
}
|