mirror of
https://github.com/RGBCube/serenity
synced 2025-07-04 01:07:35 +00:00
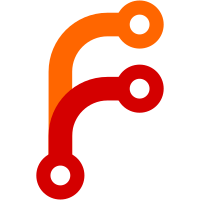
That code used the old AK::Result container, which leads to overly complicated initialization flow when trying to figure out the correct partition table type. Instead, when using the ErrorOr container the code is much simpler and more understandable.
33 lines
844 B
C++
33 lines
844 B
C++
/*
|
|
* Copyright (c) 2020-2022, Liav A. <liavalb@hotmail.co.il>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <AK/Error.h>
|
|
#include <AK/NonnullOwnPtr.h>
|
|
#include <AK/RefPtr.h>
|
|
#include <AK/Result.h>
|
|
#include <AK/Vector.h>
|
|
#include <Kernel/Storage/Partition/DiskPartition.h>
|
|
#include <Kernel/Storage/Partition/MBRPartitionTable.h>
|
|
|
|
namespace Kernel {
|
|
|
|
struct EBRPartitionHeader;
|
|
class EBRPartitionTable : public MBRPartitionTable {
|
|
public:
|
|
~EBRPartitionTable();
|
|
|
|
static ErrorOr<NonnullOwnPtr<EBRPartitionTable>> try_to_initialize(StorageDevice const&);
|
|
explicit EBRPartitionTable(StorageDevice const&);
|
|
virtual bool is_valid() const override { return m_valid; };
|
|
|
|
private:
|
|
void search_extended_partition(StorageDevice const&, MBRPartitionTable&, u64, size_t limit);
|
|
|
|
bool m_valid { false };
|
|
};
|
|
}
|