mirror of
https://github.com/RGBCube/serenity
synced 2025-05-18 20:15:08 +00:00
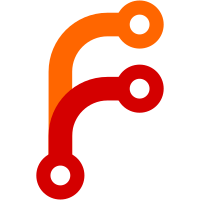
Until now, we've been treating the bottom of every line box fragment as its baseline, and just aligning all the bottoms to the bottom of the line box. That gave decent results in many cases, but was not correct. This patch starts moving towards actual baseline calculations as specified by CSS2. Note that once layout is finished with a line box, we also store the baseline of the line box in LineBox::m_baseline. This allows us to align the real baseline of display:inline-block elements with other inline content on the same line.
43 lines
1.2 KiB
C++
43 lines
1.2 KiB
C++
/*
|
|
* Copyright (c) 2018-2020, Andreas Kling <kling@serenityos.org>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <AK/Vector.h>
|
|
#include <LibWeb/Layout/LineBoxFragment.h>
|
|
|
|
namespace Web::Layout {
|
|
|
|
class LineBox {
|
|
public:
|
|
LineBox() { }
|
|
|
|
float width() const { return m_width; }
|
|
float bottom() const { return m_bottom; }
|
|
float baseline() const { return m_baseline; }
|
|
|
|
void add_fragment(Node const& layout_node, int start, int length, float leading_size, float trailing_size, float content_width, float content_height, float border_box_top, float border_box_bottom, LineBoxFragment::Type = LineBoxFragment::Type::Normal);
|
|
|
|
Vector<LineBoxFragment> const& fragments() const { return m_fragments; }
|
|
Vector<LineBoxFragment>& fragments() { return m_fragments; }
|
|
|
|
void trim_trailing_whitespace();
|
|
|
|
bool is_empty_or_ends_in_whitespace() const;
|
|
bool is_empty() const { return m_fragments.is_empty(); }
|
|
|
|
private:
|
|
friend class BlockContainer;
|
|
friend class InlineFormattingContext;
|
|
friend class LineBuilder;
|
|
|
|
Vector<LineBoxFragment> m_fragments;
|
|
float m_width { 0 };
|
|
float m_bottom { 0 };
|
|
float m_baseline { 0 };
|
|
};
|
|
|
|
}
|