mirror of
https://github.com/RGBCube/serenity
synced 2025-05-17 03:45:07 +00:00
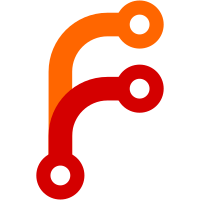
This feature had bitrotted somewhat and would trigger errors because PrimitiveStrings were "destroyed" but because of this mode they were not removed from the string cache. Even fixing that case running test-js with the options still failed in more places.
76 lines
1.6 KiB
C++
76 lines
1.6 KiB
C++
/*
|
|
* Copyright (c) 2020, Andreas Kling <kling@serenityos.org>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <AK/Format.h>
|
|
#include <AK/Forward.h>
|
|
#include <AK/Noncopyable.h>
|
|
#include <LibJS/Forward.h>
|
|
|
|
namespace JS {
|
|
|
|
class Cell {
|
|
AK_MAKE_NONCOPYABLE(Cell);
|
|
AK_MAKE_NONMOVABLE(Cell);
|
|
|
|
public:
|
|
virtual void initialize(GlobalObject&) { }
|
|
virtual ~Cell() { }
|
|
|
|
bool is_marked() const { return m_mark; }
|
|
void set_marked(bool b) { m_mark = b; }
|
|
|
|
enum class State {
|
|
Live,
|
|
Dead,
|
|
};
|
|
|
|
State state() const { return m_state; }
|
|
void set_state(State state) { m_state = state; }
|
|
|
|
virtual const char* class_name() const = 0;
|
|
|
|
class Visitor {
|
|
public:
|
|
void visit(Cell* cell)
|
|
{
|
|
if (cell)
|
|
visit_impl(*cell);
|
|
}
|
|
void visit(Value);
|
|
|
|
protected:
|
|
virtual void visit_impl(Cell&) = 0;
|
|
virtual ~Visitor() = default;
|
|
};
|
|
|
|
virtual bool is_environment() const { return false; }
|
|
virtual void visit_edges(Visitor&) { }
|
|
|
|
Heap& heap() const;
|
|
VM& vm() const;
|
|
|
|
protected:
|
|
Cell() { }
|
|
|
|
private:
|
|
bool m_mark : 1 { false };
|
|
State m_state : 7 { State::Live };
|
|
};
|
|
|
|
}
|
|
|
|
template<>
|
|
struct AK::Formatter<JS::Cell> : AK::Formatter<FormatString> {
|
|
ErrorOr<void> format(FormatBuilder& builder, JS::Cell const* cell)
|
|
{
|
|
if (!cell)
|
|
return Formatter<FormatString>::format(builder, "Cell{nullptr}");
|
|
else
|
|
return Formatter<FormatString>::format(builder, "{}({})", cell->class_name(), cell);
|
|
}
|
|
};
|