mirror of
https://github.com/RGBCube/serenity
synced 2025-05-16 19:35:08 +00:00
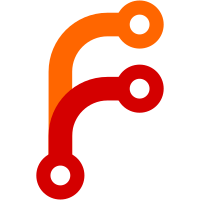
This patch adds a map of Layout::Node to FormattingState::NodeState. Instead of updating layout nodes incrementally as layout progresses through the formatting contexts, all updates are now written to the corresponding NodeState instead. At the end of layout, FormattingState::commit() is called, which transfers all the values from the NodeState objects to the Node. This will soon allow us to perform completely non-destructive layouts which don't affect the tree. Note that there are many imperfections here, and still many places where we assign to the NodeState, but later read directly from the Node instead. I'm just committing at this stage to make subsequent diffs easier to understand.
79 lines
2.6 KiB
C++
79 lines
2.6 KiB
C++
/*
|
|
* Copyright (c) 2022, Andreas Kling <kling@serenityos.org>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <AK/HashMap.h>
|
|
#include <LibGfx/Point.h>
|
|
#include <LibWeb/Layout/LineBox.h>
|
|
#include <LibWeb/Layout/Node.h>
|
|
|
|
namespace Web::Layout {
|
|
|
|
struct FormattingState {
|
|
struct NodeState {
|
|
float content_width { 0 };
|
|
float content_height { 0 };
|
|
Gfx::FloatPoint offset;
|
|
|
|
float margin_left { 0 };
|
|
float margin_right { 0 };
|
|
float margin_top { 0 };
|
|
float margin_bottom { 0 };
|
|
|
|
float border_left { 0 };
|
|
float border_right { 0 };
|
|
float border_top { 0 };
|
|
float border_bottom { 0 };
|
|
|
|
float padding_left { 0 };
|
|
float padding_right { 0 };
|
|
float padding_top { 0 };
|
|
float padding_bottom { 0 };
|
|
|
|
float offset_left { 0 };
|
|
float offset_right { 0 };
|
|
float offset_top { 0 };
|
|
float offset_bottom { 0 };
|
|
|
|
Vector<LineBox> line_boxes;
|
|
|
|
float margin_box_left() const { return margin_left + border_left + padding_left; }
|
|
float margin_box_right() const { return margin_right + border_right + padding_right; }
|
|
float margin_box_top() const { return margin_top + border_top + padding_top; }
|
|
float margin_box_bottom() const { return margin_bottom + border_bottom + padding_bottom; }
|
|
|
|
float border_box_left() const { return border_left + padding_left; }
|
|
float border_box_right() const { return border_right + padding_right; }
|
|
float border_box_top() const { return border_top + padding_top; }
|
|
float border_box_bottom() const { return border_bottom + padding_bottom; }
|
|
|
|
float border_box_width() const { return border_box_left() + content_width + border_box_right(); }
|
|
float border_box_height() const { return border_box_top() + content_height + border_box_bottom(); }
|
|
};
|
|
|
|
void commit();
|
|
|
|
NodeState& ensure(NodeWithStyleAndBoxModelMetrics const& box)
|
|
{
|
|
return *nodes.ensure(&box, [] { return make<NodeState>(); });
|
|
}
|
|
|
|
NodeState const& get(NodeWithStyleAndBoxModelMetrics const& box) const
|
|
{
|
|
if (!nodes.contains(&box))
|
|
return const_cast<FormattingState&>(*this).ensure(box);
|
|
return *nodes.get(&box).value();
|
|
}
|
|
|
|
HashMap<NodeWithStyleAndBoxModelMetrics const*, NonnullOwnPtr<NodeState>> nodes;
|
|
};
|
|
|
|
Gfx::FloatRect absolute_content_rect(Box const&, FormattingState const&);
|
|
Gfx::FloatRect margin_box_rect(Box const&, FormattingState const&);
|
|
Gfx::FloatRect margin_box_rect_in_ancestor_coordinate_space(Box const& box, Box const& ancestor_box, FormattingState const&);
|
|
|
|
}
|